C#: Create a new list from a given list of integers where each integer value is added to 2 and the result value is multiplied by 5
(Value+2) * 5 for Each Integer
Write a C# Sharp program to create a list from a given list of integers. In this program, each integer value is added to 2 and the resulting value is multiplied by 5.
Sample Solution:
C# Sharp Code:
using System; // Importing the System namespace for basic functionality
using System.Collections.Generic; // Importing the namespace for working with collections
using System.Linq; // Importing the namespace for LINQ operations
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Calling the 'test' method with a new list of integers as input
List<int> mylist = test(new List<int>(new int[] { 1, 2, 3, 4 }));
// Displaying elements of 'mylist' in the console
foreach (var i in mylist)
{
Console.Write(i.ToString() + " "); // Output each element followed by a space
}
}
// Method that takes a List of integers and performs a calculation on each element
public static List<int> test(List<int> nums)
{
// Using LINQ to perform an arithmetic operation on each element of 'nums'
// Multiplying each element by 5 times the sum of the element and 2
IEnumerable<int> e = nums.Select(x => 5 * (x + 2));
// Converting the IEnumerable 'e' to a new List and returning it
return e.ToList();
}
}
}
Sample Output:
15 20 25 30
Flowchart:
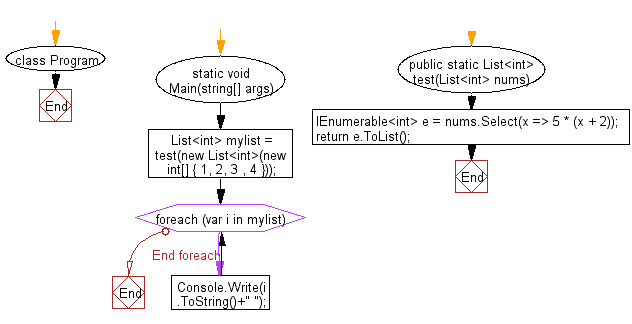
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to create a new list from a given list of strings where each element is replaced by 4 copies of the string concatenated together.
Next: Write a C# Sharp program to create a new list of the rightmost digits from a given list of positive integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.