C#: Create a new list of the rightmost digits from a given list of positive integers
C# Sharp Basic Algorithm: Exercise-146 with Solution
Rightmost Digits of Integers
Write a C# Sharp program to create a list of the rightmost digits from a given list of positive integers.
Visual Presentation:
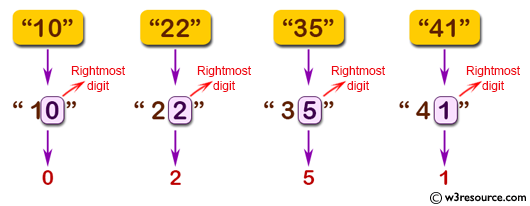
Sample Solution:
C# Sharp Code:
using System; // Importing the System namespace for basic functionality
using System.Collections.Generic; // Importing the namespace for working with collections
using System.Linq; // Importing the namespace for LINQ operations
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Calling the 'test' method with a new list of integers as input
List<int> mylist = test(new List<int>(new int[] { 10, 22, 35 , 41 }));
// Displaying elements of 'mylist' in the console
foreach(var i in mylist)
{
Console.Write(i.ToString()+" "); // Output each element followed by a space
}
}
// Method that takes a List of integers and extracts the last digit of each number
public static List<int> test(List<int> nums)
{
// Using LINQ to extract the last digit of each number in the 'nums' list
IEnumerable<int> digits = nums.Select(x => x % 10);
// Converting the IEnumerable 'digits' to a new List and returning it
return digits.ToList();
}
}
}
Sample Output:
0 2 5 1
Flowchart:
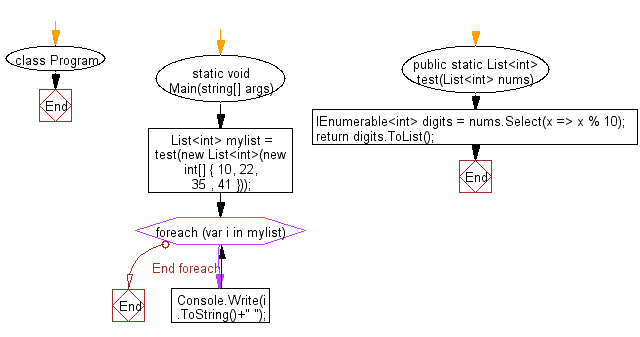
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to create a new list from a given list of integers where each integer value is added to 2 and the result value is multiplied by 5.
Next: Write a C# Sharp program to create a new list from a given list of strings where strings will be in upper case in new string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/basic-algo/csharp-basic-algorithm-exercises-146.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics