C#: Create a new list from a given list of strings where strings will be in upper case in new string
Convert Strings to Uppercase
Write a C# Sharp program to create a list from a given list of strings. Strings will be in upper case in the new string.
Visual Presentation:
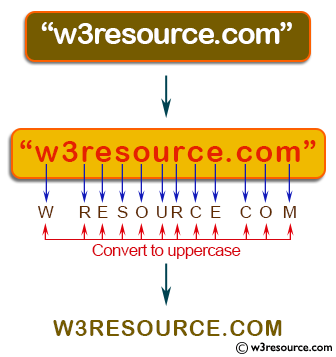
Sample Solution:
C# Sharp Code:
using System; // Importing the System namespace for basic functionality
using System.Collections.Generic; // Importing the namespace for working with collections
using System.Linq; // Importing the namespace for LINQ operations
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Calling the 'test' method with a new list of strings as input
List<string> mylist = test(new List<string>(new string[] { "Abc", "cdef", "js" , "php" }));
// Displaying elements of 'mylist' in the console
foreach(var i in mylist)
{
Console.Write(i.ToString()+" "); // Output each element followed by a space
}
}
// Method that takes a List of strings and converts each string to uppercase
public static List<string> test(List<string> str)
{
// Using LINQ to convert each string in 'str' list to uppercase
IEnumerable<string> s = str.Select(x => x.ToUpper());
// Converting the IEnumerable 's' to a new List and returning it
return s.ToList();
}
}
}
Sample Output:
ABC CDEF JS PHP
Flowchart:
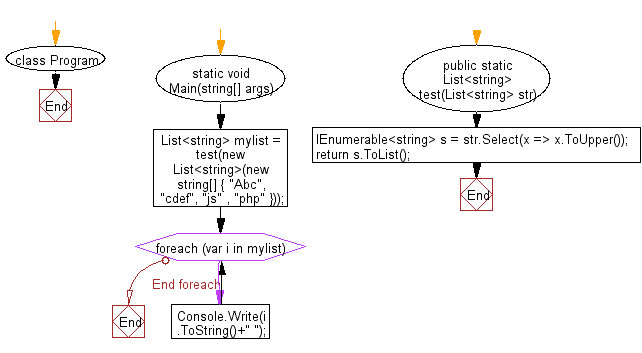
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to create a new list of the rightmost digits from a given list of positive integers.
Next: Write a C# Sharp program to remove all "a" in each string in given list of strings and return the new string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.