C# Sharp Basic Algorithm Exercises: Remove all "a" in each string in given list of strings and return the new string
Remove 'a' from Each String
Write a C# Sharp program to remove all "a"s from each string in a given list of strings and return the result string.
Visual Presentation:
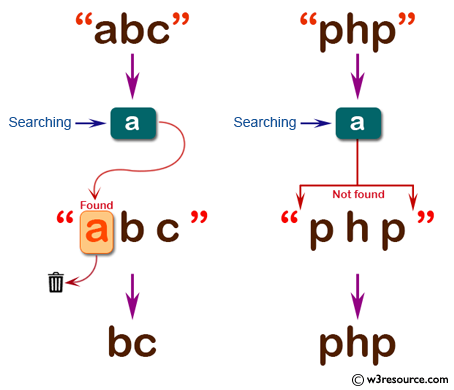
Sample Solution:
C# Sharp Code:
using System; // Importing the System namespace for basic functionality
using System.Collections.Generic; // Importing the namespace for working with collections
using System.Linq; // Importing the namespace for LINQ operations
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Calling the 'test' method with a new list of strings as input
List<string> mylist = test(new List<string>(new string[] { "abc", "cdaef", "js" , "php" }));
// Displaying elements of 'mylist' in the console
foreach(var i in mylist)
{
Console.Write(i.ToString()+" "); // Output each element followed by a space
}
}
// Method that takes a List of strings and removes all occurrences of letter 'a' from each string
public static List<string> test(List<string> str)
{
// Using LINQ to remove all occurrences of 'a' from each string in 'str' list
return str.Select(x => x.Replace("a", "")).ToList();
}
}
}
Sample Output:
bc cdef js php
Flowchart:
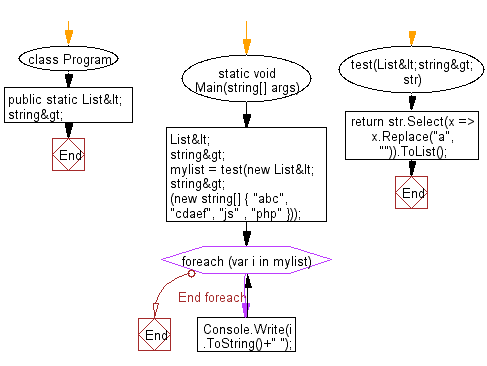
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to create a new list from a given list of strings where strings will be in upper case in new string.
Next: Write a C# Sharp program to create a new list from a given list of integers removing those values which are less than 4.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.