C#: Check whether two given integer values are in the range 20..50 inclusive
One of Two Integers in Range 20-50
Write a C# Sharp program to check whether two given integer values are in the range 20..50 inclusive. Return true if one or other is in the range, otherwise false.
Sample Solution:
C# Sharp Code:
using System;
// Namespace declaration
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Displaying the results of the 'test' method with different pairs of integers
Console.WriteLine(test(20, 84)); // Output: True
Console.WriteLine(test(14, 50)); // Output: True
Console.WriteLine(test(11, 55)); // Output: False
Console.WriteLine(test(25, 40)); // Output: True
Console.ReadLine();
}
public static bool test(int x, int y)
{
// Check if either x or y is within the range 20 to 50 inclusive
return (x >= 20 && x <= 50) || (y >= 20 && y <= 50);
}
}
}
Sample Output:
True True False True
Explanation:
- The condition checks if x is between 20 and 50 inclusive: (x >= 20 && x <= 50).
- It also checks if y is between 20 and 50 inclusive: (y >= 20 && y <= 50).
- The logical OR (||) ensures that if either of these conditions is true, the method returns true.
Test Cases and Results:
- test(20, 84) returns True because 20 is in the range 20 to 50.
- test(14, 50) returns True because 50 is in the range 20 to 50.
- test(11, 55) returns False because both 11 and 55 are outside the range of 20 to 50.
- test(25, 40) returns True because both 25 and 40 are within the range 20 to 50.
Flowchart:
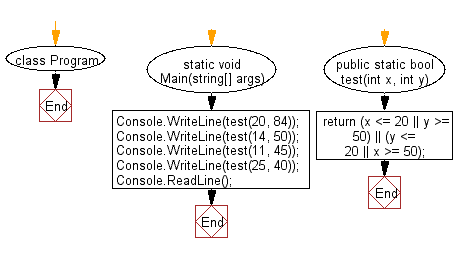
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to check whether three given integer values are in the range 20..50 inclusive. Return true if 1 or more of them are in the said range otherwise false.
Next: Write a C# Sharp program to check if a string 'yt' appears at index 1 in a given string. If it appears return a string without 'yt' otherwise return the original string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.