C#: Check whether a string 'yt' appears at index 1 in a given string
C# Sharp Basic Algorithm: Exercise-17 with Solution
Remove 'yt' at Index 1
Write a C# Sharp program to check whether a string 'yt' appears at index 1 in a given string. If it appears return a string without 'yt' otherwise return the original string.
Visual Presentation:
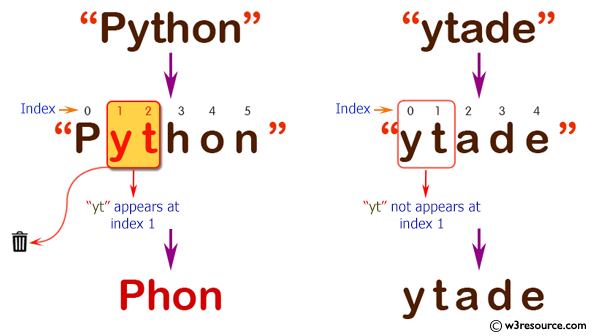
Sample Solution:
C# Sharp Code:
using System;
// Namespace declaration
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Displaying the output of the 'test' method with different strings
Console.WriteLine(test("Python")); // Output: Pyhon
Console.WriteLine(test("ytade")); // Output: yade
Console.WriteLine(test("jsues")); // Output: jsues
Console.ReadLine(); // Keeping the console window open
}
// Method to perform a specific operation on the input string
public static string test(string str)
{
// Check if substring from index 1 to index 2 (exclusive) is "yt"
// If true, remove characters at index 1 and 2 from the string; otherwise, return the original string
return str.Substring(1, 2).Equals("yt") ? str.Remove(1, 2) : str;
}
}
}
Sample Output:
Phon ytade jsues
Flowchart:
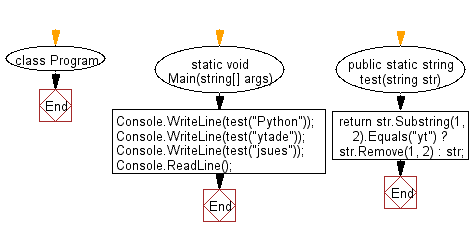
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to check whether two given integer values are in the range 20..50 inclusive. Return true if 1 or other is in the said range otherwise false.
Next: Write a C# Sharp program to check the largest number among three given integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/basic-algo/csharp-basic-algorithm-exercises-17.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics