C#: Create a new string which contain the n copies of the first 3 characters of a given string
C# Sharp Basic Algorithm: Exercise-26 with Solution
n Copies of First Three Characters
Write a C# Sharp program to create a string that is n (non-negative integer) copies of the first 3 characters of a given string. If the given string is shorter than 3, return n copies of the string.
Visual Presentation:
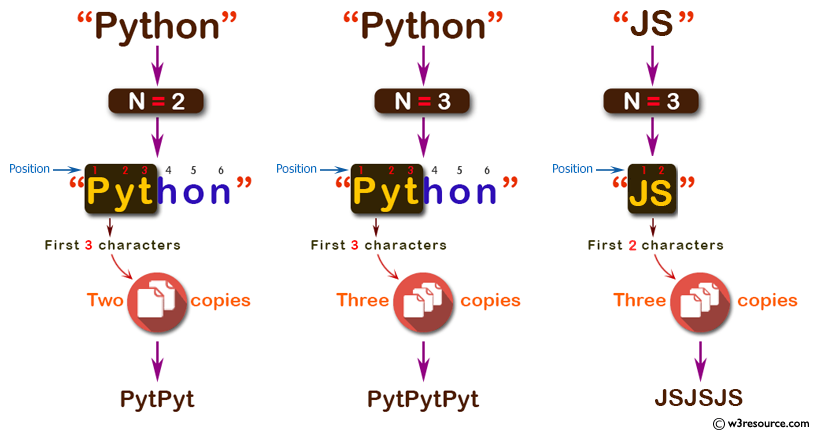
Sample Solution:-
C# Sharp Code:
using System;
// Namespace declaration
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Displaying the output of the 'test' method with different string inputs and integers
Console.WriteLine(test("Python", 2)); // Output: PytPyt
Console.WriteLine(test("Python", 3)); // Output: PytPytPyt
Console.WriteLine(test("JS", 3)); // Output: JSJSJS
Console.ReadLine(); // Keeping the console window open
}
// Method to generate a string that repeats the first few characters 'n' times
public static string test(string s, int n)
{
var result = string.Empty; // Initialize an empty string to store the resulting string
var frontOfString = 3; // Define the number of characters to repeat
// Check if the defined number of characters to repeat exceeds the length of the input string
if (frontOfString > s.Length)
frontOfString = s.Length; // Adjust the number of characters to repeat to the length of the input string
var front = s.Substring(0, frontOfString); // Extract the specified number of characters from the beginning of the input string
// Loop 'n' times to concatenate the extracted characters to the result string
for (var i = 0; i < n; i++)
{
result += front; // Concatenate the extracted characters 'n' times to the result string
}
return result; // Return the resulting concatenated string
}
}
}
Sample Output:
PytPyt PytPytPyt JSJSJS
Flowchart:
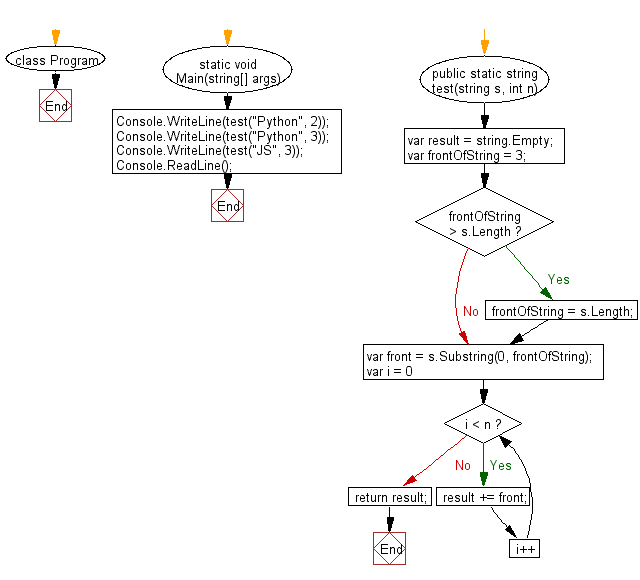
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to create a new string which is n (non-negative integer ) copies of a given string.
Next: Write a C# Sharp program to count the string "aa" in a given string and assume "aaa" contains two "aa".
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/basic-algo/csharp-basic-algorithm-exercises-26.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics