C#: Count the number of times the string 'aa' appears in a given string and assume that 'aaa' contains two 'aa'
C# Sharp Basic Algorithm: Exercise-27 with Solution
Count 'aa' in String
Write a C# Sharp program to count the number of times the string "aa" appears in a given string and assume that "aaa" contains two "aa".
Visual Presentation:
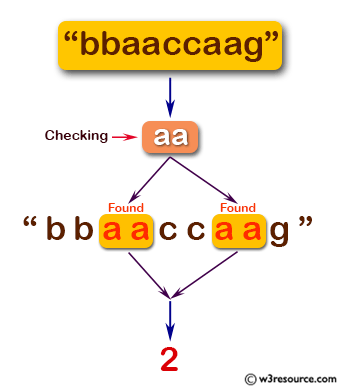
Sample Solution:-
C# Sharp Code:
using System;
// Namespace declaration
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Displaying the output of the 'test' method with different string inputs
Console.WriteLine(test("bbaaccaag")); // Output: 2
Console.WriteLine(test("jjkiaaasew")); // Output: 1
Console.WriteLine(test("JSaaakoiaa")); // Output: 2
Console.ReadLine(); // Keeping the console window open
}
// Method to count the occurrences of the substring "aa" in the given string 's'
public static int test(string s)
{
int ctr_aa = 0; // Counter to store the number of occurrences of "aa" in the string
// Loop through the string characters up to the second-to-last character
for (int i = 0; i < s.Length - 1; i++)
{
// Check if the current and next character form the substring "aa"
if (s.Substring(i, 2) == "aa")
{
ctr_aa++; // Increment the counter if "aa" is found
}
}
return ctr_aa; // Return the total count of occurrences of "aa" in the given string
}
}
}
Sample Output:
2 2 3
Flowchart:
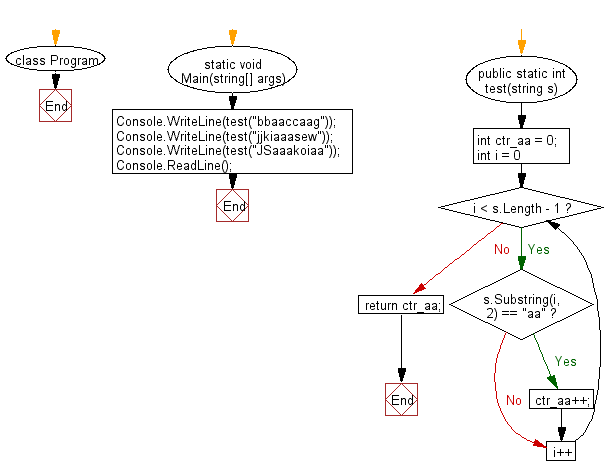
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to create a new string which is n (non-negative integer ) copies of the the first 3 characters of a given string. If the length of the given string is less than 3 then return n copies of the string.
Next: Write a C# Sharp program to check if the first appearance of "a" in a given string is immediately followed by another "a".
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/basic-algo/csharp-basic-algorithm-exercises-27.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics