C#: Check whether the first appearance of 'a' in a given string is immediately followed by another 'a'
First 'a' Followed by Another 'a'
Write a C# Sharp program to check whether the first appearance of "a" in a given string is immediately followed by another "a".
Visual Presentation:
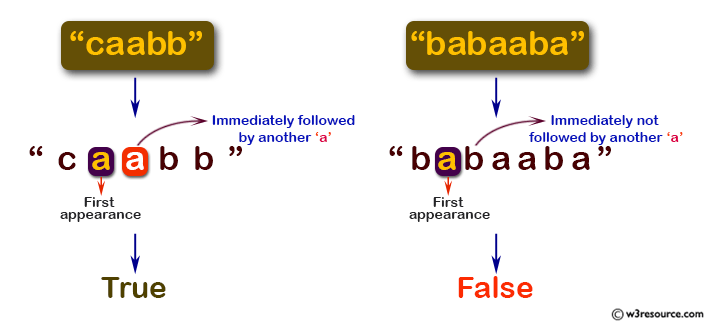
Sample Solution:-
C# Sharp Code:
using System;
// Namespace declaration
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Displaying the output of the 'test' method with different string inputs
Console.WriteLine(test("caabb")); // Output: False
Console.WriteLine(test("babaaba")); // Output: True
Console.WriteLine(test("aaaaa")); // Output: False
Console.ReadLine(); // Keeping the console window open
}
// Method to check for the presence of "aa" in the string within the first two occurrences of 'a'
public static bool test(string str)
{
var counter = 0; // Counter to track occurrences of 'a' in the string
// Loop through the characters of the string except for the last character
for (var i = 0; i < str.Length - 1; i++)
{
if (str[i].Equals('a')) // Check if the character at index 'i' is 'a'
{
counter++; // Increment the counter for each occurrence of 'a'
}
// Check if the current and next characters form the substring "aa" and the 'a' occurrences are less than 2
if (str.Substring(i, 2).Equals("aa") && counter < 2)
{
return true; // Returns true if "aa" is found within the first two 'a's
}
}
return false; // Returns false if "aa" is not found within the first two 'a's
}
}
}
Sample Output:
True False True
Flowchart:
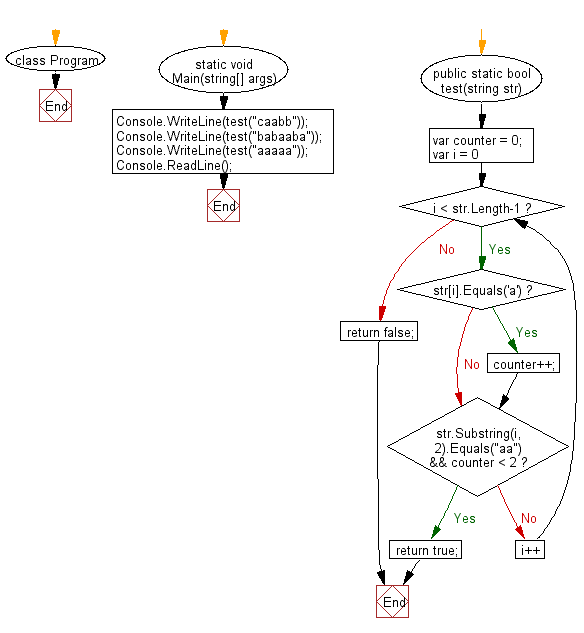
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to create a count the string "aa" in a given string and assume "aaa" contains two "aa".
Next: Write a C# Sharp program to create a new string made of every other character starting with the first from a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.