C#: Create a new string made of every other character starting with the first from a given string
C# Sharp Basic Algorithm: Exercise-29 with Solution
Every Other Character in String
Write a C# Sharp program to create a string made of every other character starting with the first in a given string.
Visual Presentation:
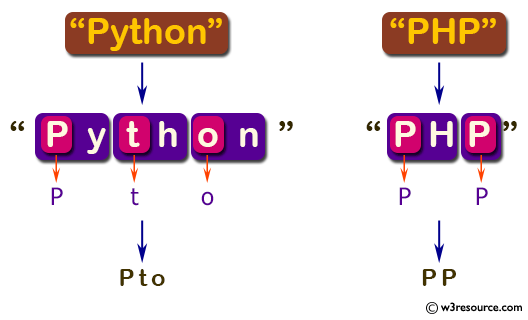
Sample Solution:-
C# Sharp Code:
using System;
// Namespace declaration
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Displaying the output of the 'test' method with different string inputs
Console.WriteLine(test("Python")); // Output: Pto
Console.WriteLine(test("PHP")); // Output: PP
Console.WriteLine(test("JS")); // Output: J
Console.ReadLine(); // Keeping the console window open
}
// Method to return a new string containing characters at even indices from the input string
public static string test(string s)
{
var result = string.Empty; // Variable to store the resulting string
// Loop through the characters of the input string
for (var i = 0; i < s.Length; i++)
{
// Check if the current index 'i' is even
if (i % 2 == 0)
{
// Append the character at index 'i' to the result string
result += s[i];
}
}
return result; // Return the string containing characters at even indices
}
}
}
Sample Output:
Pto PP J
Flowchart:
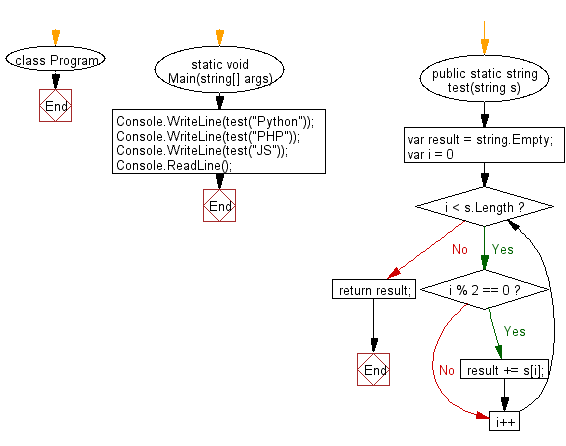
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to check if the first appearance of "a" in a given string is immediately followed by another "a".
Next: Write a C# Sharp program to create a string like "aababcabcd" from a given string "abcd".
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/basic-algo/csharp-basic-algorithm-exercises-29.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics