C#: Create a string like 'aababcabcd' from a given string 'abcd'
Cumulative Substrings
Write a C# Sharp program to create a string like 'aababcabcd' from a given string 'abcd'.
Visual Presentation:
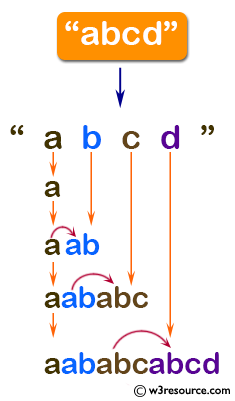
Sample Solution:-
C# Sharp Code:
using System;
// Namespace declaration
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Displaying the output of the 'test' method with different string inputs
Console.WriteLine(test("abcd")); // Output: aababcabcd
Console.WriteLine(test("abc")); // Output: aababc
Console.WriteLine(test("a")); // Output: a
Console.ReadLine(); // Keeping the console window open
}
// Method to create a new string by concatenating substrings from the input string
public static string test(string str)
{
var result = string.Empty; // Variable to store the resulting string
// Loop through the characters of the input string
for (var i = 0; i < str.Length; i++)
{
// Concatenate substrings of the input string from index 0 to 'i'
result += str.Substring(0, i + 1);
}
return result; // Return the concatenated string
}
}
}
Sample Output:
aababcabcd aababc a
Flowchart:
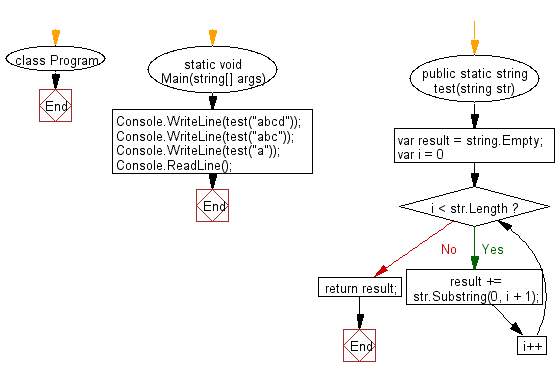
For more Practice: Solve these Related Problems:
- Write a C# program to generate a string where each character is repeated according to its position index in the original string.
- Write a C# program to build a string in the form of "a-bc-def-ghij" from a given string.
- Write a C# program to construct a cumulative string with the ASCII values of characters appended at each step.
- Write a C# program to create a string like "abacabad" where each new character extends the pattern cumulatively with some skipping rule.
Go to:
PREV : Every Other Character in String.
NEXT : Count Substring Matching Last Two Characters.
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.