C#: Check whether a triple is presents in an array of integers or not
Check Triple in Array
Write a C# Sharp program to check if a triple is present in an array of integers or not. If a value appears three times in a row in an array it is called a triple.
Visual Presentation:
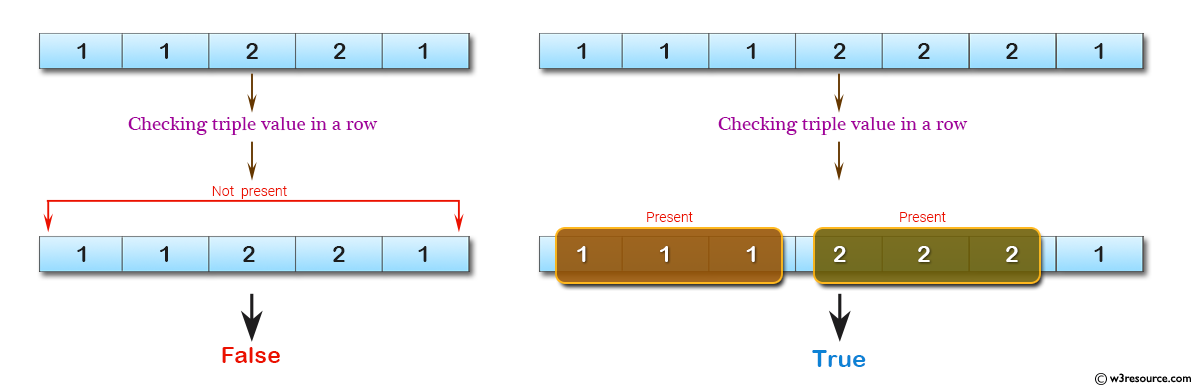
Sample Solution:-
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Calling the 'test' method with different integer arrays
Console.WriteLine(test(new[] { 1, 1, 2, 2, 1 })); // Output: false
Console.WriteLine(test(new[] { 1, 1, 2, 1, 2, 3 })); // Output: false
Console.WriteLine(test(new[] { 1, 1, 1, 2, 2, 2, 1 })); // Output: true
Console.ReadLine(); // Keeping the console window open
}
// Method to check for consecutive triplets in an integer array
public static bool test(int[] nums)
{
int arra_len = nums.Length - 1, n = 0; // Declaring variables 'arra_len' and 'n' and initializing 'arra_len' to the length of the array minus 1
for (int i = 0; i < arra_len; i++) // Loop through the array till the second-to-last element
{
n = nums[i]; // Assign the value at index 'i' to 'n'
if (n == nums[i + 1] && n == nums[i + 2]) return true; // Check if 'n' is equal to the next two consecutive elements
}
return false; // Return false if the condition is not met
}
}
}
Sample Output:
False False True
Flowchart:
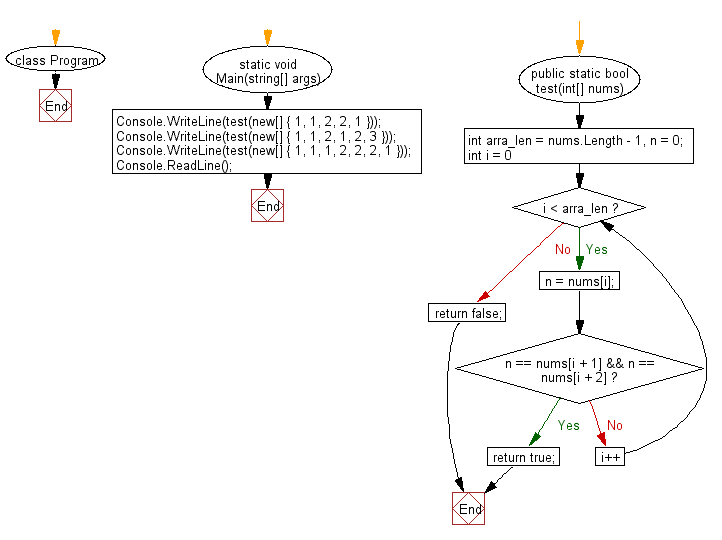
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to count the number of two 5's are next to each other in an array of integers. Also count the situation where the second 5 is actually a 6.
Next: Write a C# Sharp program to compute the sum of the two given integers. If the sum is in the range 10..20 inclusive return 30.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.