C#: Check whether a given number is within 2 of a multiple of 10
Within 2 of Multiple of 10
Write a C# Sharp program to check whether a given number is within 2 of a multiple of 10.
Sample Solution:-
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Calling the 'test' method with different integers and printing the results
Console.WriteLine(test(3)); // Output: True
Console.WriteLine(test(7)); // Output: True
Console.WriteLine(test(8)); // Output: True
Console.WriteLine(test(21)); // Output: False
Console.ReadLine(); // Keeping the console window open
}
// Method to check if the last digit of a number is less than or equal to 2 or greater than or equal to 8
public static bool test(int n)
{
// Returns true if the last digit of 'n' is less than or equal to 2 OR greater than or equal to 8
return n % 10 <= 2 || n % 10 >= 8;
}
}
}
Sample Output:
False False True True
Flowchart:
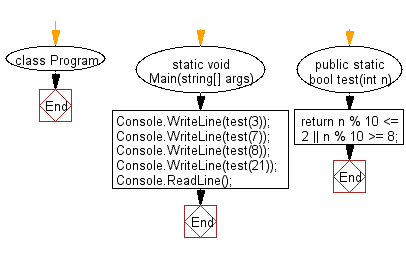
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program check if a given non-negative given number is a multiple of 3 or 7, but not both.
Next: Write a C# Sharp program to compute the sum of the two given integers. If one of the given integer value is in the range 10..20 inclusive return 18.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.