C#: Compute the sum of the two given integers
C# Sharp Basic Algorithm: Exercise-45 with Solution
Sum is 18 if One Value in 10-20 Range
Write a C# Sharp program to compute the sum of the two given integers. Return 18 if one of the given integer values is in the range of 10..20 inclusive.
visual Presentation:
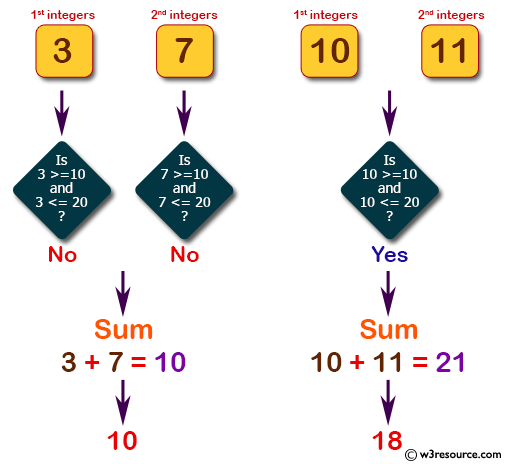
Sample Solution:-
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Calling the 'test' method with different integers and printing the results
Console.WriteLine(test(3, 7)); // Output: 18
Console.WriteLine(test(10, 11)); // Output: 18
Console.WriteLine(test(10, 20)); // Output: 18
Console.WriteLine(test(21, 220)); // Output: 241
Console.ReadLine(); // Keeping the console window open
}
// Method to determine the result based on the conditions
public static int test(int x, int y)
{
// If x is between 10 and 20 OR y is between 10 and 20, return 18; otherwise, return the sum of x and y
return (x >= 10 && x <= 20) || (y >= 10 && y <= 20) ? 18 : x + y;
}
}
}
Sample Output:
10 18 18 241
Flowchart:
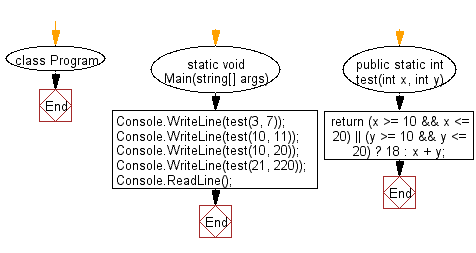
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program check if a given number is within 2 of a multiple of 10.
Next: Write a C# Sharp program to check whether a given string starts with "F" or ends with "B".
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/basic-algo/csharp-basic-algorithm-exercises-45.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics