C#: Check whether a given string starts with 'F' or ends with "B"
Fizz, Buzz, FizzBuzz
Write a C# Sharp program to check whether a given string begins with "F" or ends with "B".
If the string starts with "F" return "Fizz" and return "Buzz" if it ends with "B" If the string starts with "F" and ends with "B" return "FizzBuzz".
In other cases return the original string.
Visual Presentation:
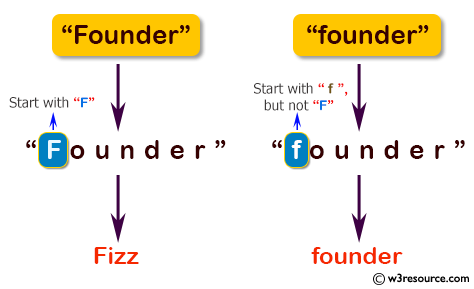
Sample Solution:-
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Calling the 'test' method with different strings and printing the results
Console.WriteLine(test("FizzBuzz")); // Output: FizzBuzz
Console.WriteLine(test("Fizz")); // Output: Fizz
Console.WriteLine(test("Buzz")); // Output: Buzz
Console.WriteLine(test("Founder")); // Output: Founder
Console.ReadLine(); // Keeping the console window open
}
// Method to determine the output based on specific conditions in the input string
public static string test(string str)
{
// Check if the string starts with "F" and ends with "B"
if (str.StartsWith("F") && str.EndsWith("B"))
{
return "FizzBuzz"; // If both conditions are true, return "FizzBuzz"
}
// Check if the string starts with "F"
else if (str.StartsWith("F"))
{
return "Fizz"; // If the string starts with "F", return "Fizz"
}
// Check if the string ends with "B"
else if (str.EndsWith("B"))
{
return "Buzz"; // If the string ends with "B", return "Buzz"
}
else
{
return str; // Return the original string if none of the conditions are met
}
}
}
}
Sample Output:
Fizz Fizz Buzz Fizz
Flowchart:
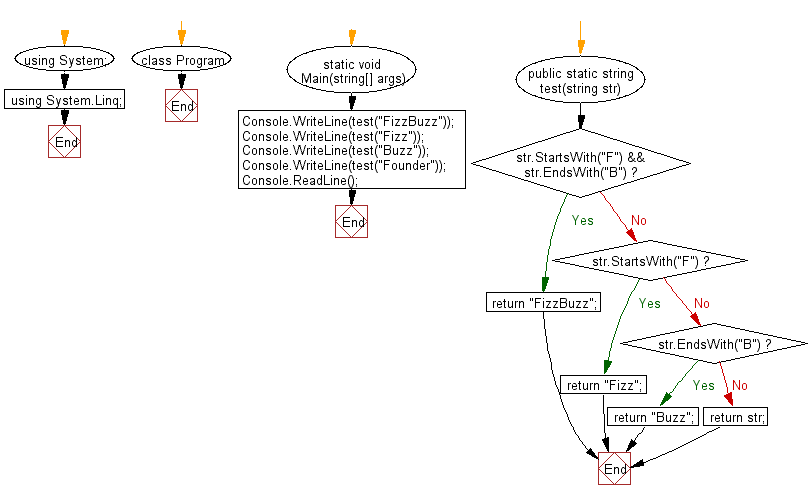
For more Practice: Solve these Related Problems:
- Write a C# program to return "Fizz" if a string contains more vowels than consonants, "Buzz" otherwise.
- Write a C# program that returns "Fizz" if a string starts and ends with the same character, otherwise "Buzz".
- Write a C# program to return "FizzBuzz" if the string contains both uppercase and lowercase letters.
- Write a C# program to return "Fizz" if the first letter is a digit and "Buzz" if the last is a digit.
Go to:
PREV : Sum is 18 if One Value in 10-20 Range.
NEXT : Sum of Two Equals Third.
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.