C#: Check whether it is possible to add two integers to get the third integer from three given integers
Sum of Two Equals Third
Write a C# Sharp program to check whether it is possible to add two integers to get the third integer from three given integers.
Visual Presentation:
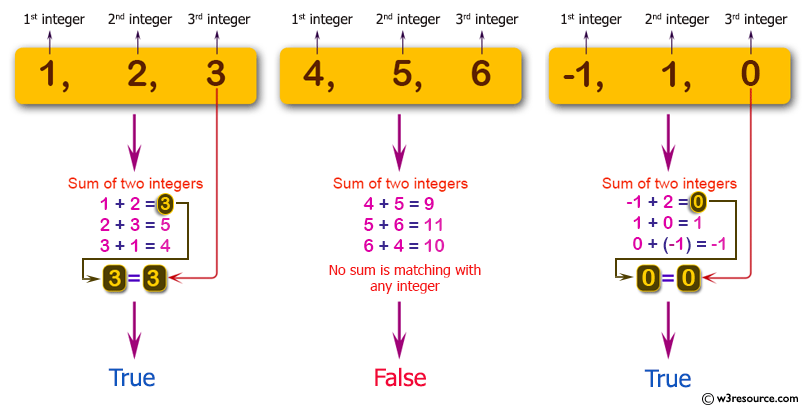
Sample Solution:-
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Calling the 'test' method with different integer values and printing the results
Console.WriteLine(test(1, 2, 3)); // Output: True
Console.WriteLine(test(4, 5, 6)); // Output: True
Console.WriteLine(test(-1, 1, 0)); // Output: True
Console.ReadLine(); // Keeping the console window open
}
// Method to check if any two numbers among three add up to the third number
public static bool test(int x, int y, int z)
{
// Returns true if any two numbers among x, y, and z add up to the third number
return x == y + z || y == x + z || z == x + y;
}
}
}
Sample Output:
True False True
Flowchart:
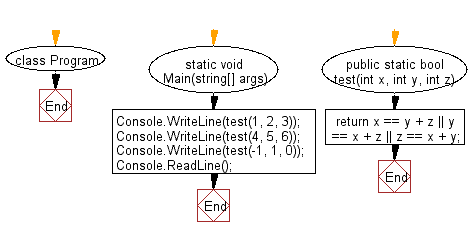
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program check whether a given string starts with "f" or ends with "b".
If the string starts with "f" return "Fizz" and return "Buzz" if it ends with "b" If the string starts with "F" and ends with "B" return "FizzBuzz".
In other cases return the original string.
Next: Write a C# Sharp program to check if y is greater than x, and z is greater than y from three given integers x,y,z.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.