C#: Check three given integers and return true if one of them is 20 or more less than one of the others
Check if One Integer 20 Less Than Another
Write a C# Sharp program to check three given integers and return true if one of them is 20 or more less than one of the others.
Visual Presentation:
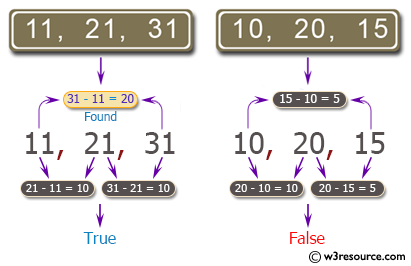
Sample Solution:-
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Printing the results of the 'test' method with different integer values
Console.WriteLine(test(11, 21, 31)); // Output: True (since the differences between all combinations of numbers are greater than or equal to 20)
Console.WriteLine(test(11, 22, 31)); // Output: False (since the difference between 11 and 22 is less than 20)
Console.WriteLine(test(10, 20, 15)); // Output: True (since the differences between all combinations of numbers are greater than or equal to 20)
Console.ReadLine(); // Keeping the console window open
}
// Method to check if the absolute difference between any two numbers among x, y, and z is greater than or equal to 20
public static bool test(int x, int y, int z)
{
// Returns true if the absolute differences between x and y, x and z, or y and z are all greater than or equal to 20
return Math.Abs(x - y) >= 20 || Math.Abs(x - z) >= 20 || Math.Abs(y - z) >= 20;
}
}
}
Sample Output:
True True False
Flowchart:
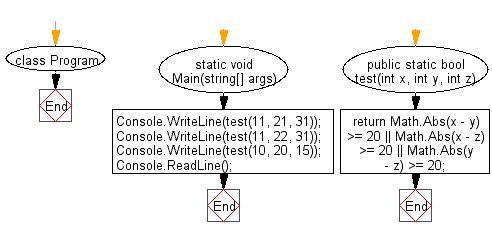
For more Practice: Solve these Related Problems:
- Write a C# program to determine if any two numbers among three differ by 20 or more and the third is not in between them.
- Write a C# program to return true if one number is exactly 20 less or more than the average of the other two.
- Write a C# program to check if the difference between the largest and smallest number is at least 20.
- Write a C# program to return true if one of the numbers is both 20 less than one and 20 more than another.
Go to:
PREV : Same Rightmost Digit in Two or More Integers.
NEXT : Largest of Two Integers or Smallest if Same Remainder by 7.
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.