C#: Find the larger from two given integers
Largest of Two Integers or Smallest if Same Remainder by 7
Write a C# Sharp program to find the largest of two given integers. However if the two integers have the same remainder when divided by 7, then return the smallest integer. If the two integers are the same, return 0.
Visual Presentation:
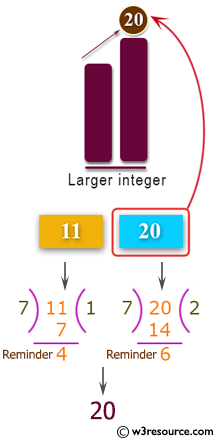
Sample Solution:-
C# Sharp Code:
using System;
using System.Linq;
namespace exercises {
// Class declaration
class Program {
// Main method - entry point of the program
static void Main(string[] args) {
// Printing the results of the 'test' method with different integer values
Console.WriteLine(test(11, 21)); // Output: 11 (since both numbers are not equal and their remainders when divided by 7 are not equal)
Console.WriteLine(test(11, 20)); // Output: 20 (since both numbers are not equal but their remainders when divided by 7 are equal)
Console.WriteLine(test(24, 10)); // Output: 24 (since both numbers are not equal and their remainders when divided by 7 are not equal)
Console.WriteLine(test(10, 10)); // Output: 0 (since both numbers are equal)
Console.ReadLine(); // Keeping the console window open
}
// Method to compare two integers and return based on certain conditions
public static int test(int x, int y) {
if (x == y) return 0; // If x is equal to y, return 0
if (x % 7 == y % 7) return (x < y) ? x : y; // If the remainders of x and y when divided by 7 are equal, return the smaller number
return (x > y) ? x : y; // If none of the above conditions are met, return the larger number
}
}
}
Sample Output:
21 20 10 0
Flowchart:
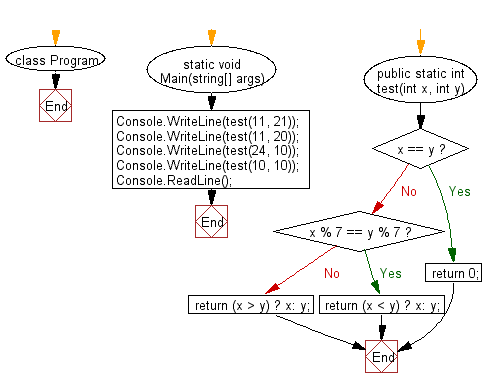
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to check three given integers and return true if one of them is 20 or more less than one of the others.
Next: Write a C# Sharp program to check two given integers, each in the range 10..99. Return true if a digit appears in both numbers, such as the 3 in 13 and 33
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.