C#: Check two given integers, each in the range 10..99
Digit Appears in Both Integers
Write a C# Sharp program to check two given integers, each in the range 10..99. Return true if a digit appears in both numbers, such as the 3 in 13 and 33
Visual Presentation:
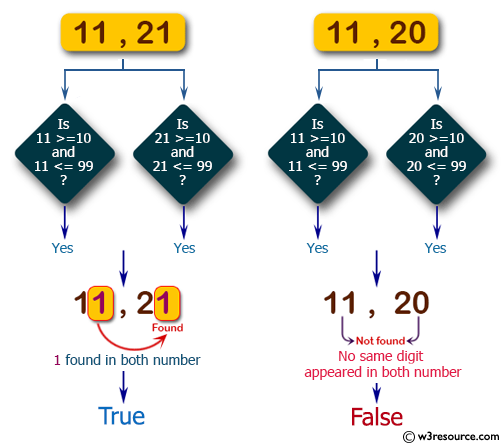
Sample Solution:-
C# Sharp Code:
using System; // Import the System namespace which contains fundamental types and base classes
using System.Linq; // Import the LINQ namespace for LINQ functionality
namespace exercises // Define a namespace called 'exercises'
{
class Program // Define a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Output the result of test function with different integer arguments
Console.WriteLine(test(11, 21)); // Test with integers 11 and 21
Console.WriteLine(test(11, 20)); // Test with integers 11 and 20
Console.WriteLine(test(10, 10)); // Test with integers 10 and 10
Console.ReadLine(); // Wait for user input before closing the console window
}
// Function definition of test function that takes two integers 'x' and 'y' and returns a boolean
public static bool test(int x, int y)
{
// Check if any of the digits in 'x' or 'y' are equal (sharing the same digit place)
return x / 10 == y / 10 || x / 10 == y % 10 || x % 10 == y / 10 || x % 10 == y % 10;
}
}
}
Sample Output:
True False True
Flowchart:
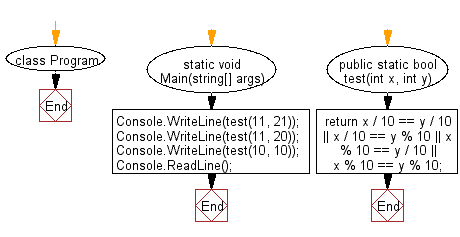
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to find the larger from two given integers. However if the two integers have the same remainder when divided by 7,then the return the smaller integer. If the two integers are the same, return 0.
Next: Write a C# Sharp program to compute the sum of two given non-negative integers x and y as long as the sum has the same number of digits as x. If the sum has more digits than x then return x without y.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.