C#: Compute the sum of two given non-negative integers x and y as long as the sum has the same number of digits as x
C# Sharp Basic Algorithm: Exercise-54 with Solution
Sum of Integers with Same Digit Count
Write a C# Sharp program to compute the sum of two given non-negative integers x and y as long as the sum has the same number of digits as x. If the sum has more digits than x return x without y.
Visual Presentation:
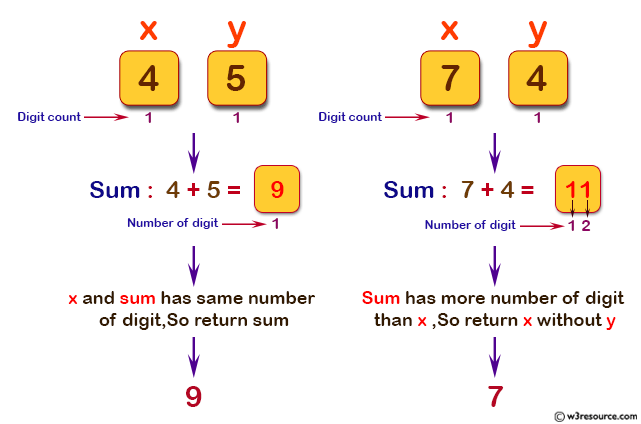
Sample Solution:-
C# Sharp Code:
using System; // Import the System namespace which contains fundamental types and base classes
using System.Linq; // Import the LINQ namespace for LINQ functionality
namespace exercises // Define a namespace called 'exercises'
{
class Program // Define a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Output the result of test function with different integer arguments
Console.WriteLine(test(4, 5)); // Test with integers 4 and 5
Console.WriteLine(test(7, 4)); // Test with integers 7 and 4
Console.WriteLine(test(10, 10)); // Test with integers 10 and 10
Console.ReadLine(); // Wait for user input before closing the console window
}
// Function definition of test function that takes two integers 'x' and 'y' and returns an integer
public static int test(int x, int y)
{
// Check if the length of the sum of 'x' and 'y' converted to a string is greater than the length of 'x' converted to a string
// If true, return 'x'; otherwise, return the sum of 'x' and 'y'
return (x + y).ToString().Length > x.ToString().Length ? x : x + y;
}
}
}
Sample Output:
9 7 20
Flowchart:
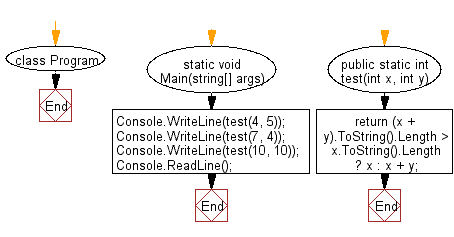
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to check two given integers, each in the range 10..99. Return true if a digit appears in both numbers, such as the 3 in 13 and 33
Next: Write a C# Sharp program to compute the sum of three given integers. If the two values are same return the third value.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/basic-algo/csharp-basic-algorithm-exercises-54.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics