C#: Compute the sum of the three given integers
Sum Ignoring 10-20 Except 13, 17
Write a C# Sharp program to compute the sum of the three given integers. All values in the range 10..20 inclusive count as 0, except 13 and 17.
Visual Presentation:
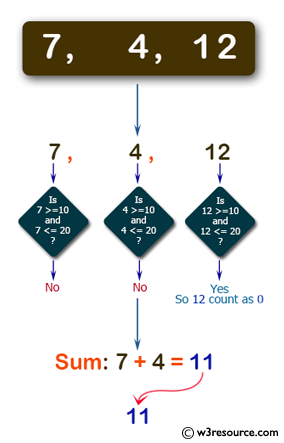
Sample Solution:-
C# Sharp Code:
using System; // Import the System namespace which contains fundamental types and base classes
using System.Collections.Generic; // Import the System.Collections.Generic namespace for collections
using System.Linq; // Import the LINQ namespace for LINQ functionality
using System.Text; // Import the System.Text namespace for string manipulation
using System.Threading.Tasks; // Import the System.Threading.Tasks namespace for asynchronous operations
namespace exercises // Define a namespace called 'exercises'
{
class Program // Define a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Output the result of test function with different sets of three integers as arguments
Console.WriteLine(test(4, 5, 7)); // Test with integers 4, 5, and 7
Console.WriteLine(test(7, 4, 12)); // Test with integers 7, 4, and 12
Console.WriteLine(test(10, 13, 12)); // Test with integers 10, 13, and 12
Console.WriteLine(test(14, 15, 16)); // Test with integers 14, 15, and 16
Console.ReadLine(); // Wait for user input before closing the console window
}
// Function definition of test function that takes three integers 'x', 'y', and 'z' and returns an integer
public static int test(int x, int y, int z)
{
// Calls the fix_num method for each integer and returns the sum of fixed values
return Program.fix_num(x) + Program.fix_num(y) + Program.fix_num(z);
}
// Private method fix_num that takes an integer 'n' and returns a fixed integer or 0
private static int fix_num(int n)
{
// Checks if 'n' falls within certain ranges and returns 0 if it does, otherwise returns 'n'
return (n > 9 && n < 13) || (n > 13 && n < 17) || (n > 17 && n < 21) ? 0 : n;
}
}
}
Sample Output:
16 11 13 0
Flowchart:
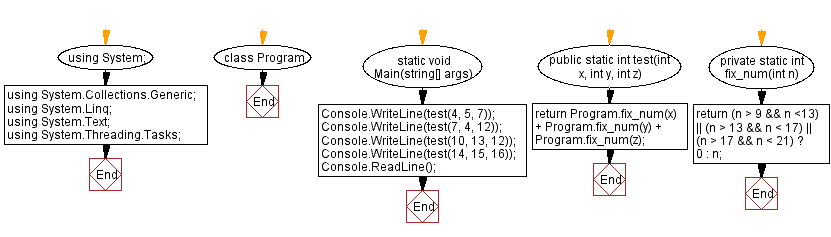
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to compute the sum of the three integers. If one of the values is 13 then do not count it and its right towards the sum.
Next: Write a C# Sharp program to check two given integers and return the value whichever value is nearest to 13 without going over. Return 0 if both numbers go over.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.