C#: Remove the character in a given position of a given string
Remove Character at Position
Write a C# Sharp program to remove the character at a given position in the string. The given position will be in the range 0..(string length -1) inclusive.
Visual Presentation:
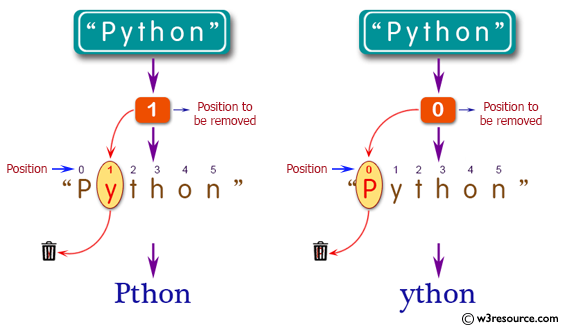
Sample Solution:
C# Sharp Code:
using System;
// Namespace declaration
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Calling the 'test' method with different strings and integers and displaying the returned values
Console.WriteLine(test("Python", 1)); // Output: "Pyton"
Console.WriteLine(test("Python", 0)); // Output: "ython"
Console.WriteLine(test("Python", 4)); // Output: "Pytho"
Console.ReadLine(); // Keeping the console window open
}
// Method to remove a character at a specified index 'n' from the input string 'str'
public static string test(string str, int n)
{
return str.Remove(n, 1); // Removing the character at index 'n' and returning the modified string
}
}
}
Sample Output:
Pthon ython Pythn
Flowchart:
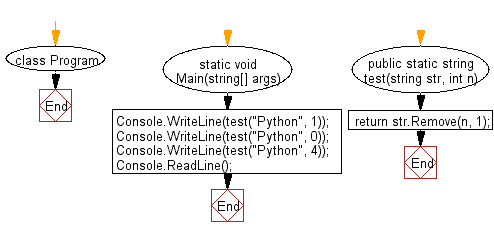
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to create a new string where "if" is added to the front of a given string. If the string already begins with 'if', return the string unchanged.
Next: Write a C# Sharp program to exchange the first and last characters in a given string and return the new string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.