C#: Exchange the first and last characters in a given string and return the new string
Exchange First and Last Characters
Write a C# Sharp program to exchange the first and last characters in a given string and return the new string.
Visual Presentation:
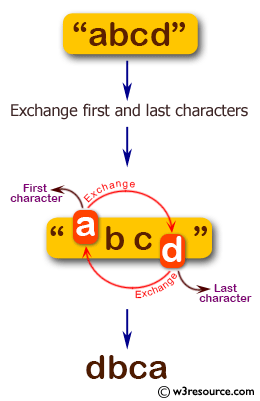
Sample Solution:
C# Sharp Code:
using System;
// Namespace declaration
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Calling the 'test' method with different strings and displaying the returned values
Console.WriteLine(test("abcd")); // Output: "dbca"
Console.WriteLine(test("a")); // Output: "a"
Console.WriteLine(test("xy")); // Output: "yx"
Console.ReadLine(); // Keeping the console window open
}
// Method to perform a specific string transformation
public static string test(string str)
{
// Checking if the length of the input string is greater than 1
// If yes, the transformation is performed; otherwise, the input string is returned as is
return str.Length > 1
// Ternary operator used to rearrange characters based on certain positions
? str.Substring(str.Length - 1) + str.Substring(1, str.Length - 2) + str.Substring(0, 1)
// Returns the input string itself if its length is 1 or 0
: str;
}
}
}
Sample Output:
dbca a yx
Flowchart:
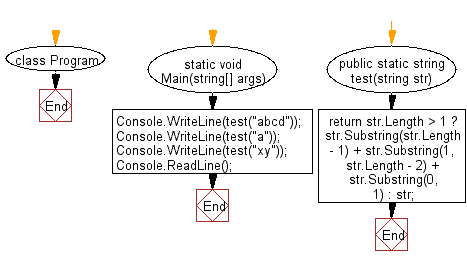
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to remove the character in a given position of a given string. The given position will be in the range 0..str.length()-1 inclusive).
Next: Write a C# Sharp program to create a new string which is 4 copies of the 2 front characters of a given string. If the given string length is less than 2 return the original string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.