C#: Create a new string which is 4 copies of the 2 front characters of a given string
Four Copies of First Two Characters
Write a C# Sharp program to create a string which is 4 copies of the 2 front characters of a given string. If the given string length is less than 2 return the original string.
Visual Presentation:
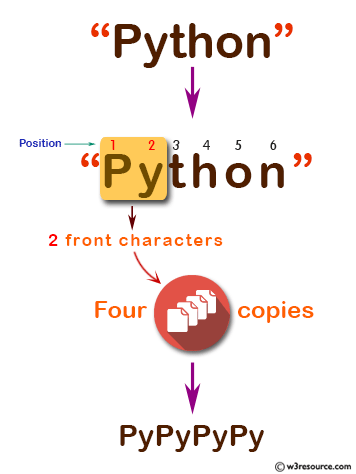
Sample Solution:
C# Sharp Code:
using System;
// Namespace declaration
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Calling the 'test' method with different strings and displaying the returned values
Console.WriteLine(test("C Sharp")); // Output: "C SharpC SharpC SharpC Sharp"
Console.WriteLine(test("JS")); // Output: "JSJSJSJS"
Console.WriteLine(test("a")); // Output: "aa"
Console.ReadLine(); // Keeping the console window open
}
// Method to perform a specific string transformation
public static string test(string str)
{
// Conditional check to determine if the length of the input string is less than 2
// If the length is less than 2, the input string is returned as is
// If the length is 2 or greater, the string is concatenated multiple times based on its first two characters
return str.Length < 2
// If the length is less than 2, returns the input string itself
? str
// If the length is 2 or more, concatenates the first two characters of the input string four times
: str.Substring(0, 2) + str.Substring(0, 2) + str.Substring(0, 2) + str.Substring(0, 2);
}
}
}
Sample Output:
C C C C JSJSJSJS a
Flowchart:
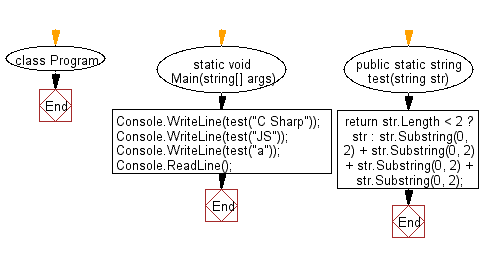
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to exchange the first and last characters in a given string and return the new string.
Next: Write a C# Sharp program to create a new string with the last char added at the front and back of a given string of length 1 or more.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.