C#: Create a new string taking the first character from a given string and the last character from another given string
First and Last Char of Two Strings
Write a C# Sharp program to create a string by taking the first character from a string and the last character from another string. If a string's length is 0, use '#' as its missing character.
Visual Presentation:
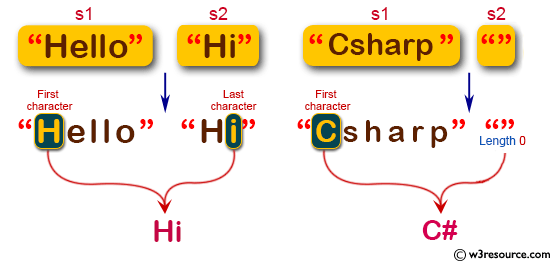
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Output the results of the test function with different strings as arguments
Console.WriteLine(test("Hello", "Hi")); // Pass "Hello" and "Hi" as arguments to the test function
Console.WriteLine(test("Python", "PHP")); // Pass "Python" and "PHP" as arguments to the test function
Console.WriteLine(test("JS", "JS")); // Pass "JS" and "JS" as arguments to the test function
Console.WriteLine(test("Csharp", "")); // Pass "Csharp" and an empty string as arguments to the test function
Console.ReadLine(); // Wait for user input before closing the console window
}
// Function definition of the test function that takes two strings 's1' and 's2' and returns a string
public static string test(string s1, string s2)
{
string lastChars = String.Empty; // Initialize an empty string 'lastChars'
if (s1.Length > 0) // Check if the length of 's1' is greater than 0
{
lastChars += s1.Substring(0, 1); // If so, append the first character of 's1' to 'lastChars'
}
else
{
lastChars += "#"; // If 's1' is empty, append "#" to 'lastChars'
}
if (s2.Length > 0) // Check if the length of 's2' is greater than 0
{
lastChars += s2.Substring(s2.Length - 1); // If so, append the last character of 's2' to 'lastChars'
}
else
{
lastChars += "#"; // If 's2' is empty, append "#" to 'lastChars'
}
return lastChars; // Return the concatenated string 'lastChars'
}
}
}
Sample Output:
Hi PP JS C#
Flowchart:
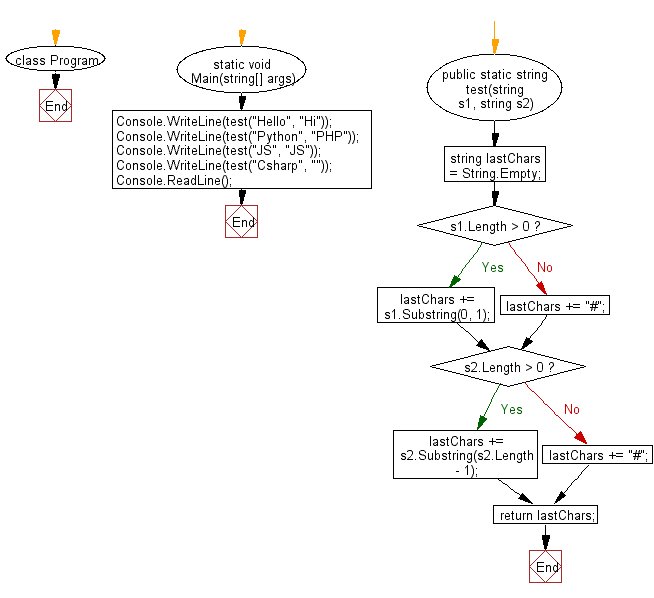
Go to:
PREV : First Two Characters with Missing '#' if Short.
NEXT : Combine Strings Removing Duplicates.
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.