C#: Combine two given strings. If there are any double character in new string then omit one character
Combine Strings Removing Duplicates
Write a C# Sharp program to combine two given strings (lowercase). If there are any double characters in the string, omit one character.
Visual Presentation:
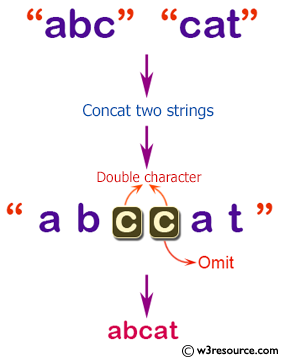
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Output the results of the test function with different strings as arguments
Console.WriteLine(test("abc", "cat")); // Pass "abc" and "cat" as arguments to the test function
Console.WriteLine(test("python", "php")); // Pass "python" and "php" as arguments to the test function
Console.WriteLine(test("php", "php")); // Pass "php" and "php" as arguments to the test function
Console.ReadLine(); // Wait for user input before closing the console window
}
// Function definition of the test function that takes two strings 's1' and 's2' and returns a string
public static string test(string s1, string s2)
{
// Check if 's1' is an empty string
if (s1.Length < 1)
{
return s2; // If 's1' is empty, return 's2'
}
// Check if 's2' is an empty string
if (s2.Length < 1)
{
return s1; // If 's2' is empty, return 's1'
}
// Check if the last character of 's1' does not match the first character of 's2'
// If true, concatenate 's1' and 's2'; otherwise, concatenate 's1' and 's2' excluding the first character of 's2'
return !s1.EndsWith(s2.Substring(0, 1)) ? s1 + s2 : s1 + s2.Substring(1);
}
}
}
Sample Output:
abcat pythonphp phphp
Flowchart:
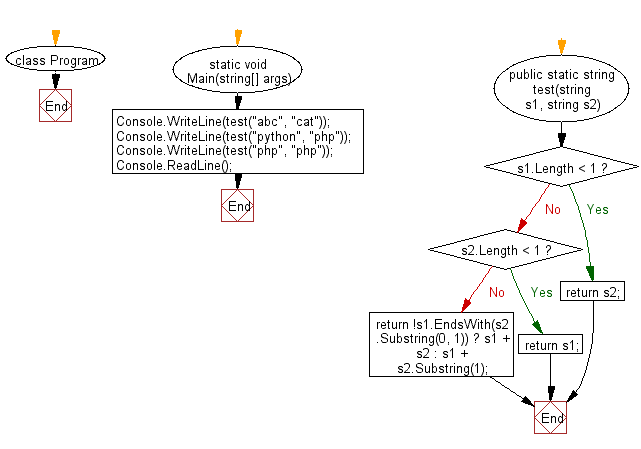
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to create a new string taking the first character from a given string and the last character from another given string. If the length of any given string is 0, use '#' as its missing character.
Next: Write a C# Sharp program to create a new string from a given string after swapping last two characters.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.