C#: Create a new string from a given string after swapping last two characters
Swap Last Two Characters
Write a C# Sharp program to create a new string from a given string after swapping the last two characters.
Visual Presentation:
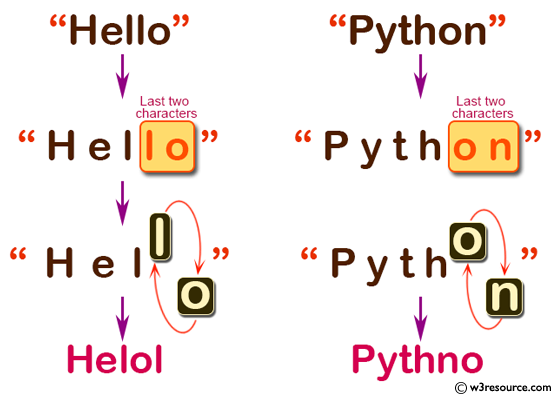
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Output the results of the test function with different strings as arguments
Console.WriteLine(test("Hello")); // Pass "Hello" to the test function and display the result
Console.WriteLine(test("Python")); // Pass "Python" to the test function and display the result
Console.WriteLine(test("PHP")); // Pass "PHP" to the test function and display the result
Console.WriteLine(test("JS")); // Pass "JS" to the test function and display the result
Console.WriteLine(test("C")); // Pass "C" to the test function and display the result
Console.ReadLine(); // Wait for user input before closing the console window
}
// Function definition of the test function that takes a string 's1' as input and returns a string
public static string test(string s1)
{
// Check if the length of 's1' is greater than 1
if (s1.Length > 1)
{
// If 's1' has a length greater than 1, rearrange the characters and return the modified string
return s1.Substring(0, s1.Length - 2) + s1[s1.Length - 1] + s1[s1.Length - 2];
}
else
{
// If 's1' has a length of 1 or less, return 's1' as it is
return s1;
}
}
}
}
Sample Output:
Helol Pythno PPH SJ C
Flowchart:
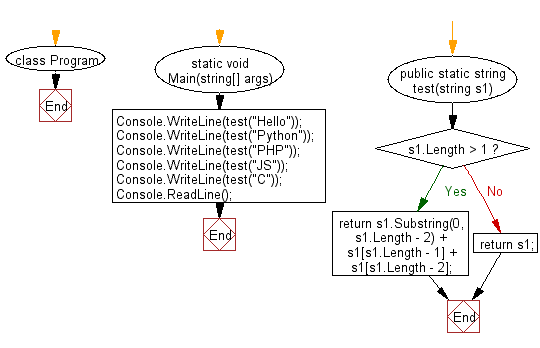
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to combine two given strings. If there are any double character in new string then omit one character.
Next: Write a C# Sharp program to check if a given string begins with "abc" or "xyz". If the string begins with "abc" or "xyz" return "abc" or "xyz" otherwise return the empty string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.