C#: Check whether a given string begins with "abc" or "xyz"
Starts with 'abc' or 'xyz'
Write a C# Sharp program to check if a given string begins with 'abc' or 'xyz'. If the string begins with 'abc' or 'xyz' return 'abc' or 'xyz' otherwise return an empty string.
visual Presentation:
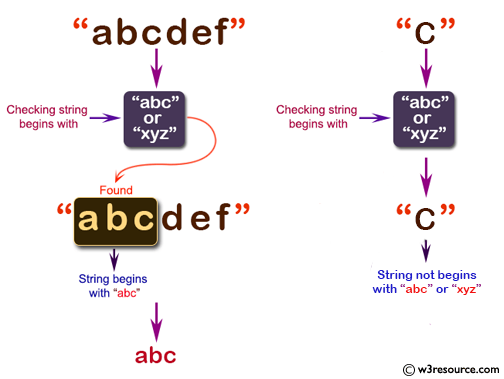
Sample Solution:-
C# Sharp Code:
using System; // Import the System namespace which contains fundamental types and base classes
namespace exercises // Define a namespace called 'exercises'
{
class Program // Define a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
Console.WriteLine(test("abc")); // Output the result of test function with "abc" as an argument
Console.WriteLine(test("abcdef")); // Output the result of test function with "abcdef" as an argument
Console.WriteLine(test("C")); // Output the result of test function with "C" as an argument
Console.WriteLine(test("xyz")); // Output the result of test function with "xyz" as an argument
Console.WriteLine(test("xyzsder")); // Output the result of test function with "xyzsder" as an argument
Console.ReadLine(); // Wait for user input before closing the console window
}
// Function definition of test function that takes a string argument 's1' and returns a string
public static string test(string s1)
{
if (s1.StartsWith("abc")) // Check if 's1' starts with "abc"
{
return "abc"; // If true, return "abc"
}
else if (s1.StartsWith("xyz")) // Check if 's1' starts with "xyz"
{
return "xyz"; // If true, return "xyz"
}
else
{
return String.Empty; // If 's1' doesn't start with "abc" or "xyz", return an empty string
}
}
}
}
Sample Output:
abc abc xyz xyz
Flowchart:
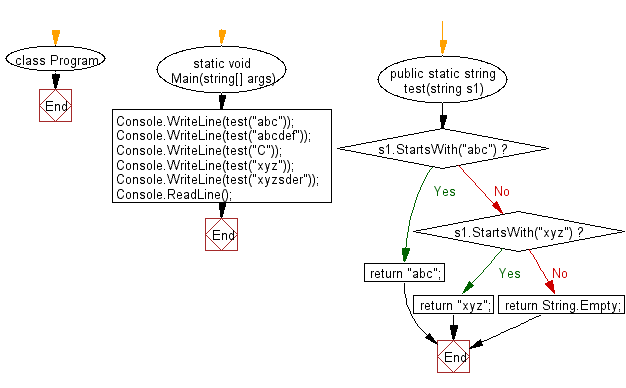
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to create a new string from a given string after swapping last two characters.
Next: Write a C# Sharp program to check whether the first two characters and last two characters of a given string are same.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.