C#: Check whether the first two characters and last two characters of a given string are same
First Two and Last Two Characters Same
Write a C# Sharp program to check whether the first two characters and the last two characters of a given string are the same.
Visual Presentation:
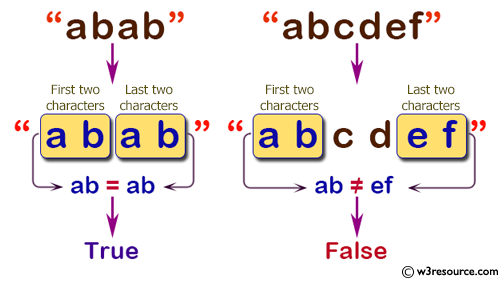
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Output the results of the test function with different strings as arguments
Console.WriteLine(test("abab")); // Pass "abab" to the test function and display the result
Console.WriteLine(test("abcdef")); // Pass "abcdef" to the test function and display the result
Console.WriteLine(test("xyzsderxy")); // Pass "xyzsderxy" to the test function and display the result
Console.ReadLine(); // Wait for user input before closing the console window
}
// Function definition of the test function that takes a string 's1' as input and returns a boolean
public static bool test(string s1)
{
// Compare the substring of the first 2 characters with the substring of the last 2 characters of 's1'
return s1.Substring(0, 2) == s1.Substring(s1.Length - 2);
}
}
}
Sample Output:
True False True
Flowchart:
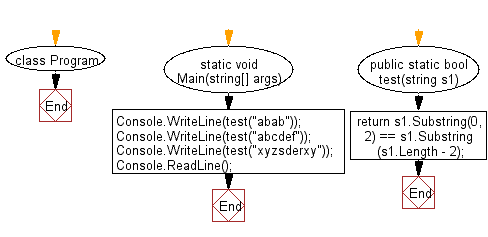
Go to:
PREV : Starts with 'abc' or 'xyz'.
NEXT : Combine Strings After Adjusting Lengths.
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.