C#: Check two given arrays of integers of length 1 or more and return true if they have the same first element or they have the same last element
Same First or Last Element in Two Arrays
Write a C# Sharp program to check two given arrays of integers of length 1 or more. Return true if they have the same first element or the same last element.
Visul Presentation:
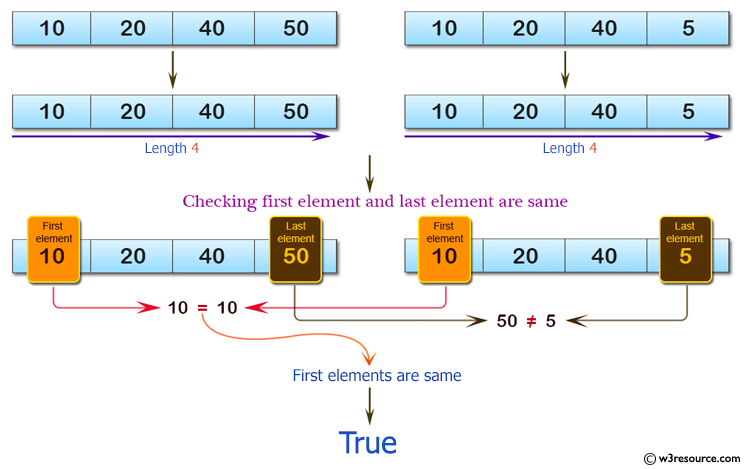
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Output the results of the 'test' function with different pairs of integer arrays as arguments
Console.WriteLine(test(new[] { 10, 20, 40, 50 }, new[] { 10, 20, 40, 50 })); // Calling test method with arrays [10, 20, 40, 50] and [10, 20, 40, 50] and displaying the result
Console.WriteLine(test(new[] { 10, 20, 40, 50 }, new[] { 10, 20, 40, 5 })); // Calling test method with arrays [10, 20, 40, 50] and [10, 20, 40, 5] and displaying the result
Console.WriteLine(test(new[] { 10, 20, 40, 50 }, new[] { 1, 20, 40, 5 })); // Calling test method with arrays [10, 20, 40, 50] and [1, 20, 40, 5] and displaying the result
Console.ReadLine(); // Waiting for user input before closing the console window
}
// Method to check if the first element of one array matches the first element of another array
// or if the last element of one array matches the last element of another array
public static bool test(int[] a1, int[] a2)
{
// Returns true if the first element of 'a1' matches the first element of 'a2'
// or if the last element of 'a1' matches the last element of 'a2'
return a1[0] == a2[0] || a1[a1.Length - 1] == a2[a2.Length - 1];
}
}
}
Sample Output:
True True False
Flowchart:
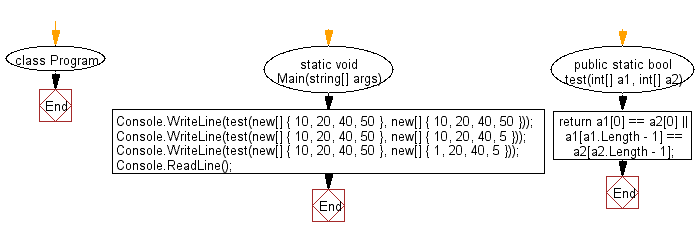
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to check a given array of integers of length 1 or more and return true if the first element and the last element are equal in the given array.
Next: Write a C# Sharp program to compute the sum of the elements of an given array of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.