C#: Compute the sum of the elements of an given array of integers
Sum of Array Elements
Write a C# Sharp program to compute the sum of the elements of an array of integers.
Visual Presentation:
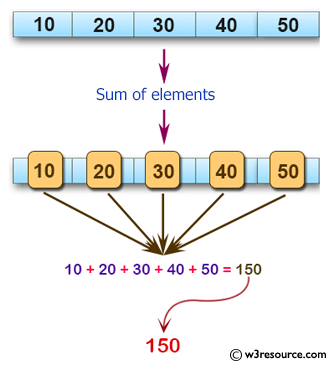
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Output the result of the 'test' function with different integer arrays as arguments
Console.WriteLine(test(new[] { 10, 20, 30, 40, 50 })); // Calling test method with array [10, 20, 30, 40, 50] and displaying the result
Console.WriteLine(test(new[] { 10, 20, -30, -40, 50 })); // Calling test method with array [10, 20, -30, -40, 50] and displaying the result
}
// Method to calculate the sum of the first five elements of an integer array
public static int test(int[] a1)
{
// Return the sum of the first five elements of the array 'a1'
return a1[0] + a1[1] + a1[2] + a1[3] + a1[4];
}
}
}
Sample Output:
150 10
Flowchart:
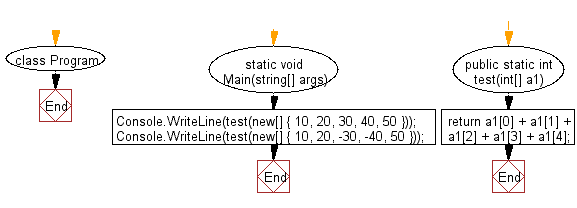
Go to:
PREV : Same First or Last Element in Two Arrays.
NEXT : Rotate Array Left.
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.