C#: rotate the elements of a given array of integers in left direction and return the new array
Rotate Array Left
Write a C# Sharp program to rotate the elements of a given array of integers (length 4 ) in the left direction and return the array.
Test Data: 90, 30, 50, 40
Sample Output: Rotated array: 30 50 40 90
Test Data: -40, 10, -20, -10
Sample Output: Rotated array: 10 -20 -10 -40
Visual Presentation:
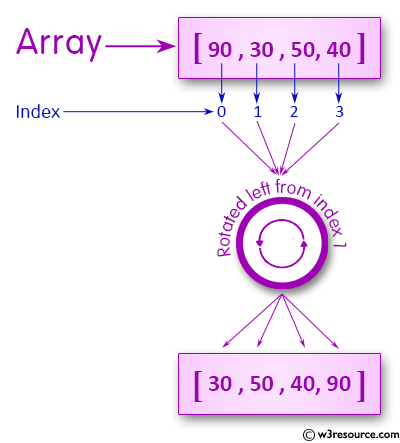
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Calling the 'test' method with an integer array as an argument and assigning the result to the 'item' array
int[] item = test(new[] { 10, 20, -30, -40 });
// Outputting text to indicate the start of displaying the rotated array
Console.Write("Rotated array: ");
// Looping through each element of the 'item' array and displaying them
foreach(var i in item)
{
Console.Write(i.ToString()+" "); // Displaying each element of the 'item' array separated by a space
}
}
// Method to rotate an integer array by one position to the left
public static int[] test(int[] a1)
{
// Returning a new array with elements rotated by one position to the left
return new int[] { a1[1], a1[2], a1[3], a1[0] };
}
}
}
Sample Output:
Rotated array: 20 -30 -40 10
Flowchart:
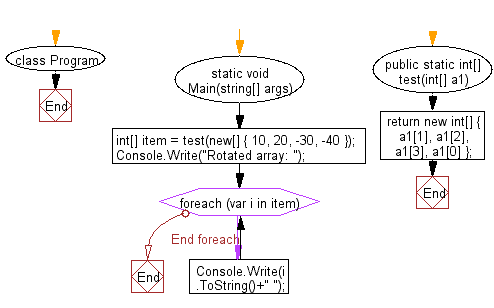
Go to:
PREV : Sum of Array Elements.
NEXT : Rotate Array Left.
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.