C#: Program to ask the user for his age and print a massage
C# Sharp Basic: Exercise-11 with Solution
Print Age Message
Write a C# Sharp program that takes an age (for example 20) as input and prints something like "You look older than 20".
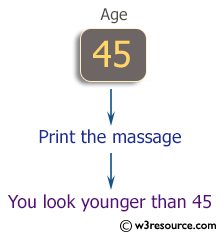
Sample Solution:
C# Sharp Code:
using System;
// This is the beginning of the Exercise11 class
public class Exercise11
{
// This is the main method where the program execution starts
public static void Main()
{
int age; // Variable to store the user's age
// Prompting the user to enter their age
Console.Write("Enter your age ");
// Reading the age entered by the user and converting it to an integer
age = Convert.ToInt32(Console.ReadLine());
// Displaying a message indicating that the user looks younger than their entered age
Console.Write("You look younger than {0} ", age);
}
}
Sample Output:
Enter your age 45 You look younger than 45
Flowchart:
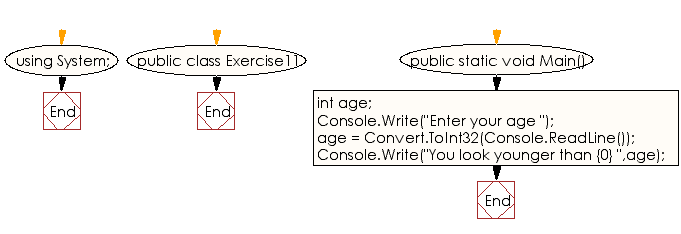
C# Sharp Code Editor:
Previous: Write a C# Sharp program to that takes three numbers(x,y,z) as input and print the output of (x+y)·z and x·y + y·z.
Next: Write a C# program to that takes a number as input and display it four times in a row (separated by blank spaces), and then four times in the next row, with no separation. You should do it two times: Use Console. Write and then use {0}
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/basic/csharp-basic-exercise-11.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics