C#: Create an array containing the middle elements of three arrays of integers
Middle Elements of Three Arrays
Write a C# program to create an new array, containing the middle elements of three arrays (each length 3) of integers.
Pictorial Presentation:
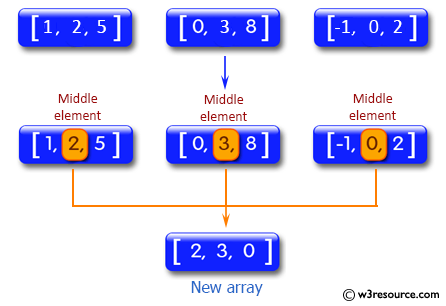
Sample Solution:
C# Sharp Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
public class Exercise52
{
public static void Main()
{
// Initializing arrays
int[] array1 = {1, 2, 5};
Console.WriteLine("\nArray1: [{0}]", string.Join(", ", array1));
int[] array2 = {0, 3, 8};
Console.WriteLine("\nArray2: [{0}]", string.Join(", ", array2));
int[] array3 = {-1, 0, 2};
Console.WriteLine("\nArray3: [{0}]", string.Join(", ", array3));
// Creating a new array by selecting elements from respective positions of array1, array2, and array3
int[] new_array = { array1[1], array2[1], array3[1] };
Console.WriteLine("\nNew array: [{0}]", string.Join(", ", new_array));
}
}
Sample Output:
Array1: [1, 2, 5] Array2: [0, 3, 8] Array3: [-1, 0, 2] New array: [2, 3, 0]
Flowchart:
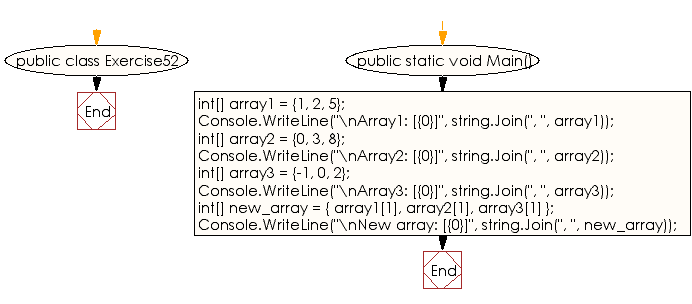
For more Practice: Solve these Related Problems:
- Write a C# program to extract the middle values from five arrays (each length 3) and return them in reverse order.
- Write a C# program to combine middle values of three arrays and find their average.
- Write a C# program to create a new array using the middle elements of three arrays only if all middle elements are even.
- Write a C# program to check whether the middle values of three arrays form an arithmetic sequence.
Go to:
PREV : Max of First and Last in Array.
NEXT : Check Odd Number in Array.
C# Sharp Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.