C#: Check if an array contains an odd number
Check Odd Number in Array
Write a C# program to check if an array contains an odd number.
Pictorial Presentation:
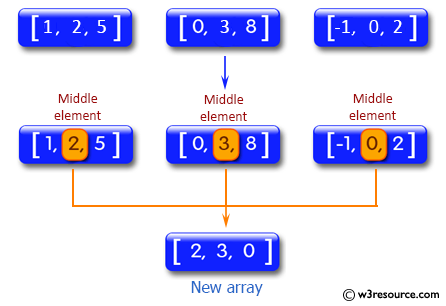
Sample Solution:
C# Sharp Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
public class Exercise53
{
public static void Main()
{
// Initializing an array of integers
int[] nums = {2, 4, 7, 8, 6};
// Displaying the original array
Console.WriteLine("\nOriginal array: [{0}]", string.Join(", ", nums));
// Checking if the array contains an odd number and displaying the result
Console.WriteLine("Check if an array contains an odd number? " + even_odd(nums));
}
// Function to check if the array contains an odd number
public static bool even_odd(int[] nums)
{
// Iterating through each element of the array
foreach (var n in nums)
{
// Checking if the element is odd
if (n % 2 != 0)
return true; // If an odd number is found, return true
}
return false; // If no odd number is found, return false
}
}
Sample Output:
Original array: [2, 4, 7, 8, 6] Check if an array contains an odd number? True
Flowchart:
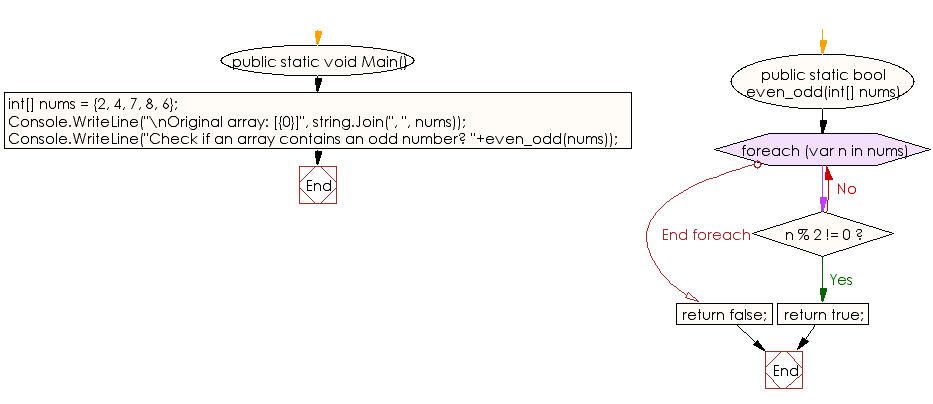
C# Sharp Code Editor:
Previous: Write a C# program to create an new array, containing the middle elements of three arrays (each length 3) of integers.
Next: Write a C# program to get the century from a year.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.