C#: Check if a given number present in an array of numbers
C# Sharp Basic: Exercise-63 with Solution
Check Number in Array
Write a C# program to check if a given number is present in an array of numbers.
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
// Main method where the program execution begins
static void Main(string[] args)
{
int[] nums = { 1, 3, 5, 7, 9 };
int n = 6;
// Calls the test function and prints the result
Console.WriteLine(test(nums, n));
n = 3;
// Calls the test function again with a different value of n and prints the result
Console.WriteLine(test(nums, n));
}
// Function to test if an array contains a specific element
public static bool test(int[] arra, int n)
{
// Uses the Contains method of LINQ to check if the array contains the given number 'n'
return arra.Contains(n);
}
}
}
Sample Output:
False True
Flowchart:
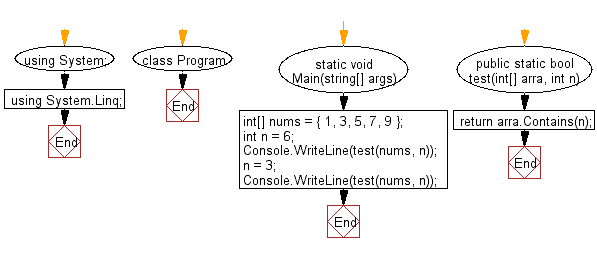
Sample Solution-1:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
// Main method where the program execution begins
static void Main(string[] args)
{
int[] nums = { 1, 3, 5, 7, 9 };
int n = 6;
// Calls the test function and prints the result
Console.WriteLine(test(nums, n));
n = 3;
// Calls the test function again with a different value of n and prints the result
Console.WriteLine(test(nums, n));
}
// Function to test if an array contains a specific element using a foreach loop
public static bool test(int[] arra, int n)
{
// Loop through each element in the array
foreach (int item in arra)
{
// Check if the current element matches the given number 'n'
if (item == n)
return true; // If found, return true immediately
}
return false; // If not found after checking all elements, return false
}
}
}
Sample Output:
False True
Flowchart:
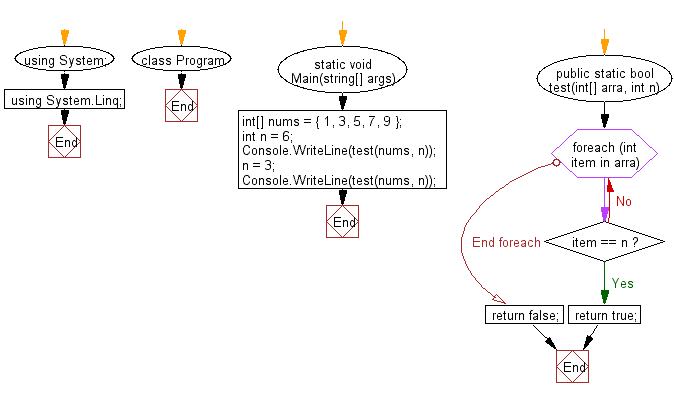
C# Sharp Code Editor:
Previous: Write a C# program to reverse the strings contained in each pair of matching parentheses in a given string and also remove the parentheses within the given string.
Next: Write a C# Sharp program to get the file name (including extension) from a given path.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/basic/csharp-basic-exercise-63.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics