C#: File name (including extension) from a given path
Get File Name from Path
Write a C# Sharp program to get the file name (including extension) from a given path.
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
// Main method where the program execution begins
static void Main(string[] args)
{
string file_path;
// Initialize file_path variable with a file path and call the test function
file_path = "c:/csharp/ex/test.cpp";
Console.WriteLine(test(file_path)); // Output: test.cpp
// Change file_path and call the test function again
file_path = "c:/movies/abc.mp4";
Console.WriteLine(test(file_path)); // Output: abc.mp4
// Change file_path to a different path and call the test function
file_path = "test.txt";
Console.WriteLine(test(file_path)); // Output: test.txt
}
// Function to extract the file name from a file path
public static string test(string file_path)
{
// Split the file path using '/' as the separator and extract the last part (file name)
return file_path.Split('/').Last();
}
}
}
Sample Output:
test.cpp abc.mp4 test.txt
Flowchart:
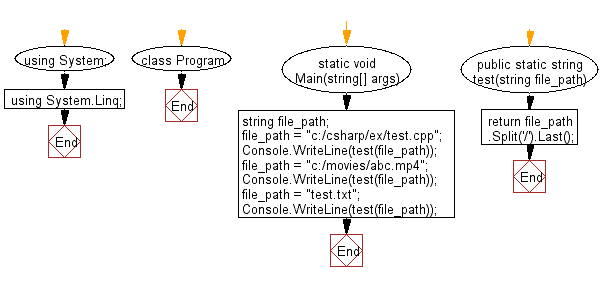
Sample Solution-1:
C# Sharp Code:
using System;
using System.IO; // Importing the System.IO namespace for file path operations
namespace exercises
{
class Program
{
// Main method where the program execution begins
static void Main(string[] args)
{
string file_path;
// Initialize file_path variable with a file path and call the test function
file_path = "c:/csharp/ex/test.cpp";
Console.WriteLine(test(file_path)); // Output: test.cpp
// Change file_path and call the test function again
file_path = "c:/movies/abc.mp4";
Console.WriteLine(test(file_path)); // Output: abc.mp4
// Change file_path to a different path and call the test function
file_path = "test.txt";
Console.WriteLine(test(file_path)); // Output: test.txt
}
// Function to extract the file name from a file path using Path.GetFileName method
public static string test(string file_path)
{
// Using Path.GetFileName method to extract the file name from the file path
return Path.GetFileName(file_path);
}
}
}
Sample Output:
test.cpp abc.mp4 test.txt
Flowchart:
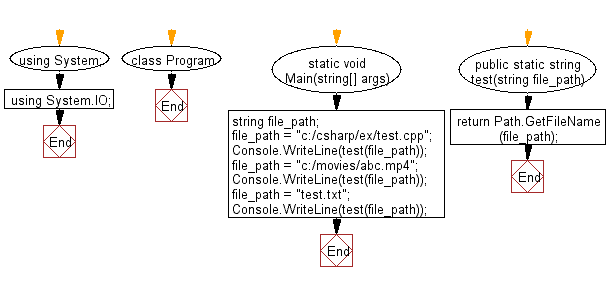
C# Sharp Code Editor:
Previous: Write a C# program to check if a given number present in an array of numbers.
Next: Write a C# Sharp program to multiply all of elements of a given array of numbers by the array length.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.