C#: Multiply all of elements of a given array of numbers by the array length
C# Sharp Basic: Exercise-65 with Solution
Multiply Array Elements by Length
Write a C# Sharp program to multiply all elements of a given array of numbers by array length.
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
// Main method where the program execution begins
static void Main(string[] args)
{
int[] nums = { 1, 3, 5, 7, 9 };
// Call the test function and store the returned array in new_nums
int[] new_nums = test(nums);
// Print each element of the new_nums array using Array.ForEach and Console.WriteLine
Array.ForEach(new_nums, Console.WriteLine);
}
// Function to multiply each element of the input array by the array length
public static int[] test(int[] nums)
{
// Get the length of the input array
var arr_len = nums.Length;
// Using LINQ's Select method to multiply each element by the array length
// and converting it back to an array using ToArray()
return nums.Select(el => el * arr_len).ToArray();
}
}
}
Sample Output:
5 15 25 35 45
Flowchart:
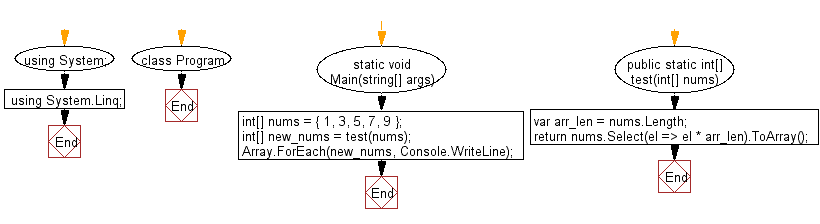
Sample Solution-1:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
// Main method where the program execution begins
static void Main(string[] args)
{
int[] nums = { 1, 3, 5, 7, 9 };
// Call the test function and store the returned array in new_nums
int[] new_nums = test(nums);
// Print each element of the new_nums array using Array.ForEach and Console.WriteLine
Array.ForEach(new_nums, Console.WriteLine);
}
// Function to multiply each element of the input array by the array length
public static int[] test(int[] nums)
{
// Loop through each element of the input array
for (int i = 0; i < nums.Length; i++)
{
nums[i] *= nums.Length; // Multiply each element by the length of the array
}
return nums; // Return the modified array
}
}
}
Sample Output:
5 15 25 35 45
Flowchart:
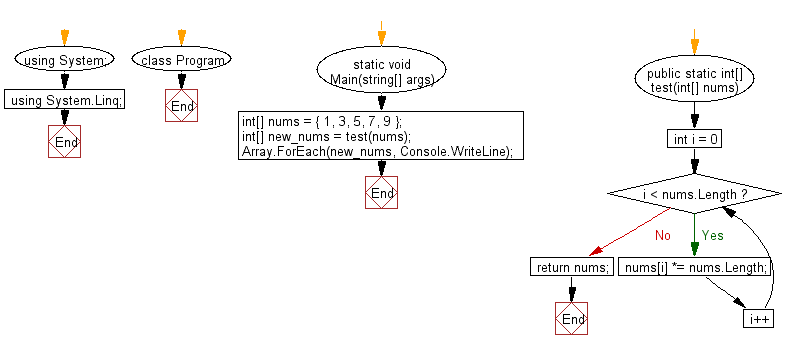
C# Sharp Code Editor:
Previous: Write a C# Sharp program to get the file name (including extension) from a given path.
Next: Write a C# Sharp program to find the minimum value from two given two numbers, represented as string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/basic/csharp-basic-exercise-65.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics