C#: Find the minimum value from two given two numbers, represented as string
Min Value from Two String Numbers
Write a C# Sharp program to find the minimum value from two numbers given to you, represented as a string.
Sample Solution:
C# Sharp Code:
using System;
namespace exercises
{
class Program
{
// Main method where the program execution begins
static void Main(string[] args)
{
// Calls the test function with two string arguments and prints the result
Console.WriteLine(test("12", "43"));
}
// Function to compare two string representations of numbers and return the smaller one
public static string test(string strn1, string strn2)
{
// Parsing the string representations of numbers to integers using Int32.Parse
// Comparing the parsed integers using a ternary operator and returning the smaller string
return Int32.Parse(strn1) > Int32.Parse(strn2) ? strn2 : strn1;
}
}
}
Sample Output:
12
Flowchart:
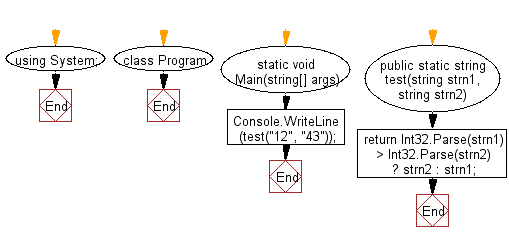
For more Practice: Solve these Related Problems:
- Write a C# program to compare three string-represented numbers and return the smallest one.
- Write a C# program to compare two numeric strings, ignoring leading zeros, and return the smaller one.
- Write a C# program to compare two string numbers and return the smaller one only if they are both integers.
- Write a C# program to validate and find the minimum of two string numbers even if one contains a decimal point.
Go to:
PREV : Multiply Array Elements by Length.
NEXT : Coded String Conversion.
C# Sharp Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.