C#: Count a specified character (both cases) in a given string
Count Specific Character in String
Write a C# Sharp program to count a specified character (both cases) in a given string.
Sample Solution:
C# Sharp Code:
using System;
namespace exercises
{
class Program
{
// Main method where the program execution begins
static void Main(string[] args)
{
// Calls the test function with different string arguments and characters, and prints the results
Console.WriteLine(test("PHP Exercises", 'E', 'e')); // Output: 2
Console.WriteLine(test("Latest News, Breaking News LIVE", 'A', 'a')); // Output: 3
}
// Function to count occurrences of characters in a string (case-insensitive)
public static int test(string str1, char uc, char lc)
{
// Using Split method to count occurrences of characters (case-insensitive)
// Splitting the string using provided uppercase and lowercase characters as separators,
// then getting the count of splits minus 1 (as the count of splits equals occurrences)
return str1.Split(uc, lc).Length - 1;
}
}
}
Sample Output:
3 2
Flowchart:
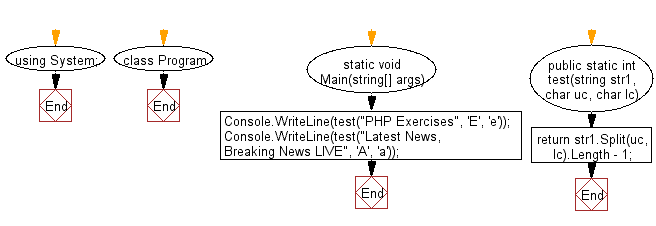
Sample Solution-1:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
// Main method where the program execution begins
static void Main(string[] args)
{
// Calls the test function with different string arguments and characters, and prints the results
Console.WriteLine(test("PHP Exercises", 'E', 'e')); // Output: 2
Console.WriteLine(test("Latest News, Breaking News LIVE", 'A', 'a')); // Output: 3
}
// Function to count occurrences of specified characters in a string (case-insensitive)
public static int test(string str1, char uc, char lc)
{
// Using LINQ's Where method to filter characters that match the specified uppercase or lowercase characters,
// then using Count method to count the occurrences of matching characters
return str1.Where(ch => (ch == lc || ch == uc)).Count();
}
}
}
Sample Output:
3 2
Flowchart:
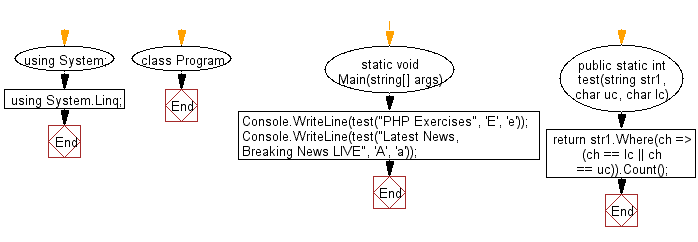
For more Practice: Solve these Related Problems:
- Write a C# program to count how many vowels appear in a given string.
- Write a C# program to count occurrences of every character in a string (case-insensitive).
- Write a C# program to count all alphanumeric characters excluding punctuation in a string.
- Write a C# program to count how many times the most frequent character appears in a string.
Go to:
PREV : Coded String Conversion.
NEXT : Check All Uppercase or Lowercase.
C# Sharp Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.