C#: Check if a given string contains only lowercase or uppercase characters
Check All Uppercase or Lowercase
Write a C# Sharp program to check if a given string contains only lowercase or uppercase characters.
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
// Main method where the program execution begins
static void Main(string[] args)
{
// Calls the test function with different string arguments and prints the results
Console.WriteLine(test("PHP")); // Output: True
Console.WriteLine(test("python")); // Output: True
Console.WriteLine(test("JavaScript")); // Output: False
}
// Function to check if a string contains both uppercase and lowercase characters
public static bool test(string str1)
{
// Checking if the input string is equal to its uppercase version OR its lowercase version
// If the string is either completely uppercase or completely lowercase, it returns true, otherwise false
return str1 == str1.ToUpper() || str1 == str1.ToLower();
}
}
}
Sample Output:
True True False
Flowchart:
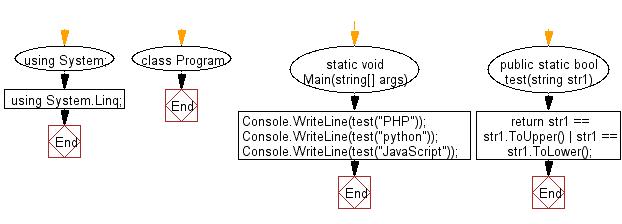
Sample Solution-1:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
// Main method where the program execution begins
static void Main(string[] args)
{
// Calls the test function with different string arguments and prints the results
Console.WriteLine(test("PHP")); // Output: True
Console.WriteLine(test("python")); // Output: True
Console.WriteLine(test("JavaScript")); // Output: False
}
// Function to check if a string contains characters in either all uppercase or all lowercase
public static bool test(string str1)
{
// Checking if the input string is entirely in uppercase OR entirely in lowercase
// If the string is either completely uppercase or completely lowercase, it returns true, otherwise false
return str1 == str1.ToUpper() || str1 == str1.ToLower();
}
}
}
Sample Output:
True True False
Flowchart:
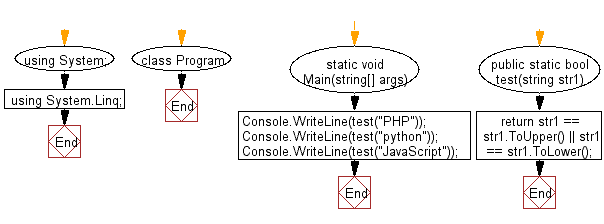
C# Sharp Code Editor:
Previous: Write a C# Sharp program to count a specified character (both cases) in a given string.
Next: Write a C# Sharp program to remove the first and last elements from a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.