C#: Remove the first and last elements from a given string
Remove First and Last Characters
Write a C# Sharp program to remove the first and last elements from a given string.
Sample Solution:
C# Sharp Code:
using System;
namespace exercises
{
class Program
{
// Main method where the program execution begins
static void Main(string[] args)
{
// Displays original strings and the result after removing first and last elements
Console.WriteLine("Original string: PHP");
Console.WriteLine("After removing first and last elements: " + test("PHP"));
Console.WriteLine("Original string: Python");
Console.WriteLine("After removing first and last elements: " + test("Python"));
Console.WriteLine("Original string: JavaScript");
Console.WriteLine("After removing first and last elements: " + test("JavaScript"));
}
// Function to remove the first and last characters of a string
public static string test(string str1)
{
// Using conditional (ternary) operator to check if string length is greater than 2
// If string length is greater than 2, it returns the substring excluding the first and last characters
// If string length is less than or equal to 2, it returns the original string
return str1.Length > 2 ? str1.Substring(1, str1.Length - 2) : str1;
}
}
}
Sample Output:
Original string: PHP After removing first and last elements: H Original string: Python After removing first and last elements: ytho Original string: JavaScript After removing first and last elements: avaScrip
Flowchart:
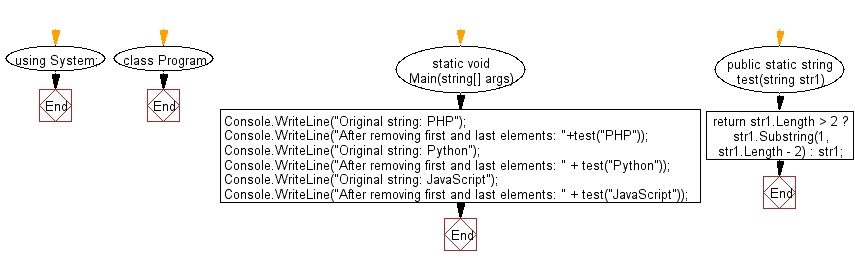
Sample Solution-1:
C# Sharp Code:
using System;
namespace exercises
{
class Program
{
// Main method where the program execution begins
static void Main(string[] args)
{
// Displays original strings and the result after removing first and last elements
Console.WriteLine("Original string: PHP");
Console.WriteLine("After removing first and last elements: " + test("PHP"));
Console.WriteLine("Original string: Python");
Console.WriteLine("After removing first and last elements: " + test("Python"));
Console.WriteLine("Original string: JavaScript");
Console.WriteLine("After removing first and last elements: " + test("JavaScript"));
}
// Function to remove the first and last characters of a string
public static string test(string str1)
{
// Using conditional (ternary) operator to check if string length is less than or equal to 2
// If string length is less than or equal to 2, it returns the original string
// If string length is greater than 2, it returns the substring excluding the first and last characters
return str1.Length <= 2 ? str1 : str1.Substring(1, str1.Length - 2);
}
}
}
Sample Output:
Original string: PHP After removing first and last elements: H Original string: Python After removing first and last elements: ytho Original string: JavaScript After removing first and last elements: avaScrip
Flowchart:
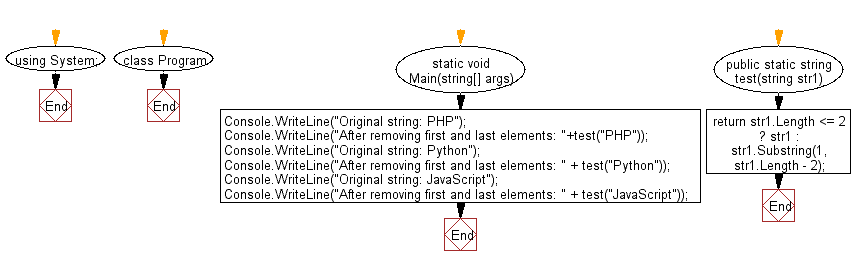
C# Sharp Code Editor:
Previous: Write a C# Sharp program to check if a given string contains only lowercase or uppercase characters.
Next: Write a C# Sharp program to check if a given string contains two similar consecutive letters.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.