C#: Check whether a given word is plural or not
Check Word Plural
Singular means only one and plural means more than one. In order to make a noun plural, it is usually only necessary to add s.
Write a C# Sharp program to check whether a word is plural or not.
Sample Solution:
C# Sharp Code:
using System;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Displaying whether 'Exercise' is considered plural by calling the test method
Console.WriteLine("Is 'Exercise' plural? " + test("Exercise"));
// Displaying whether 'Exercises' is considered plural by calling the test method
Console.WriteLine("Is 'Exercises' plural? " + test("Exercises"));
// Displaying whether 'Books' is considered plural by calling the test method
Console.WriteLine("Is 'Books' plural? " + test("Books"));
// Displaying whether 'Book' is considered plural by calling the test method
Console.WriteLine("Is 'Book' plural? " + test("Book"));
}
// Method to test if a word is plural based on its ending
public static bool test(string word)
{
// Checking if the word ends with the letter 's'
return word.EndsWith("s");
}
}
}
Sample Output:
Is 'Exercise' is plural? False Is 'Exercises' is plural? True Is 'Books' is plural? True Is 'Book' is plural? False
Flowchart:
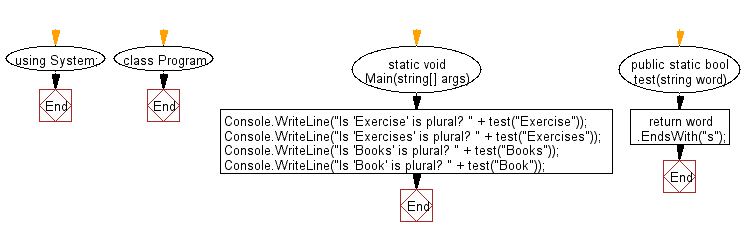
Sample Solution-1:
C# Sharp Code:
using System;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Displaying whether 'Exercise' is considered plural by calling the test method
Console.WriteLine("Is 'Exercise' plural? " + test("Exercise"));
// Displaying whether 'Exercises' is considered plural by calling the test method
Console.WriteLine("Is 'Exercises' plural? " + test("Exercises"));
// Displaying whether 'Books' is considered plural by calling the test method
Console.WriteLine("Is 'Books' plural? " + test("Books"));
// Displaying whether 'Book' is considered plural by calling the test method
Console.WriteLine("Is 'Book' plural? " + test("Book"));
}
// Method to test if a word is plural based on its ending
public static bool test(string word)
{
int word_len = word.Length; // Getting the length of the input word
return (word[word_len - 1] == 's') == true; // Checking if the last character of the word is 's'
}
}
}
Sample Output:
Is 'Exercise' is plural? False Is 'Exercises' is plural? True Is 'Books' is plural? True Is 'Book' is plural? False
Flowchart:
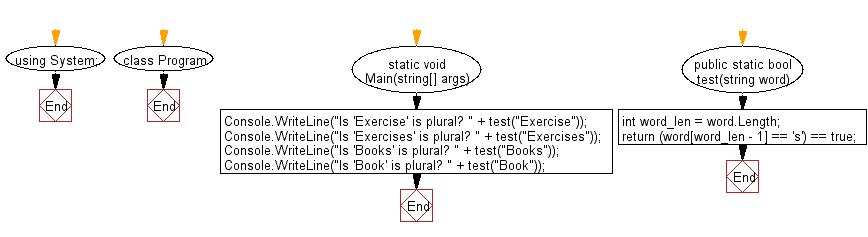
For more Practice: Solve these Related Problems:
- Write a C# program to check if a word is singular, plural, or ambiguous using custom suffix rules.
- Write a C# program to pluralize a given singular noun, accounting for common irregular nouns.
- Write a C# program that takes a sentence and returns only the plural words.
- Write a C# program to identify plural words in a string based on simple and complex pluralization patterns.
Go to:
PREV : Get ASCII Value of Character.
NEXT : Sum of Squares in Array.
C# Sharp Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.