C#: Find sum of squares of elements of a given array of numbers
Sum of Squares in Array
Write a C# Sharp program to find the sum of squares of elements in a given array of integers.
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Array containing integers
int[] nums = { 1, 2, 3 };
// Displaying the sum of squares of elements in the array by calling the test method
Console.WriteLine("Sum of squares of elements of the said array: " + test(nums));
// Another array containing integers, including negative numbers
int[] nums1 = { -2, 0, 3, 4 };
// Displaying the sum of squares of elements in the second array by calling the test method
Console.WriteLine("Sum of squares of elements of the said array: " + test(nums1));
}
// Method to calculate the sum of squares of elements in an array
public static int test(int[] nums)
{
// Using LINQ's Sum method along with a lambda expression to calculate the sum of squares
return nums.Sum(n => n * n);
}
}
}
Sample Output:
Sum of squares of elements of the said array: 14 Sum of squares of elements of the said array: 29
Flowchart:
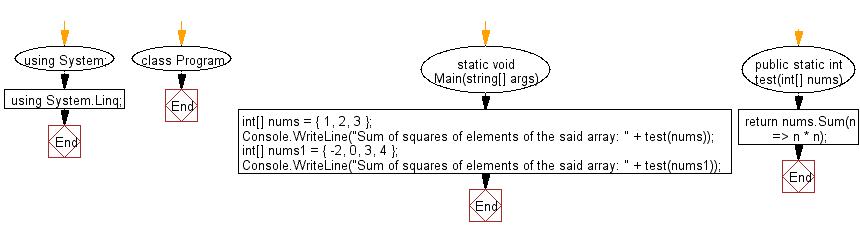
Sample Solution-1:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Array containing integers
int[] nums = { 1, 2, 3 };
// Displaying the sum of squares of elements in the array by calling the test method
Console.WriteLine("Sum of squares of elements of the said array: " + test(nums));
// Another array containing integers, including negative numbers
int[] nums1 = { -2, 0, 3, 4 };
// Displaying the sum of squares of elements in the second array by calling the test method
Console.WriteLine("Sum of squares of elements of the said array: " + test(nums1));
}
// Method to calculate the sum of squares of elements in an array using a for loop
public static int test(int[] nums)
{
int squaresSum = 0; // Variable to hold the sum of squares
// Looping through the elements of the array
for (int i = 0; i < nums.Length; i++)
{
// Calculating the square of each element and adding it to the sum
squaresSum = squaresSum + (int)Math.Pow(nums[i], 2);
}
return squaresSum; // Returning the sum of squares
}
}
}
Sample Output:
Sum of squares of elements of the said array: 14 Sum of squares of elements of the said array: 29
Flowchart:
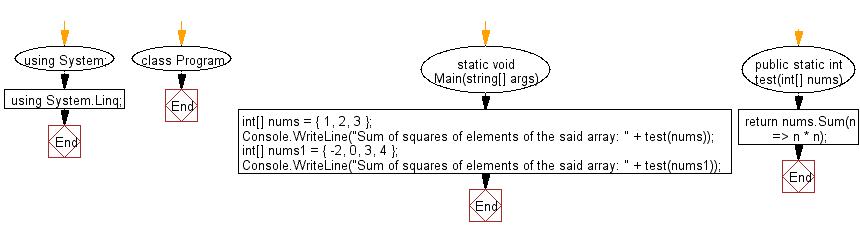
For more Practice: Solve these Related Problems:
- Write a C# program to compute the sum of squares of even numbers in an integer array.
- Write a C# program to find the difference between the square of sums and the sum of squares of an array.
- Write a C# program to compute the sum of squares of elements excluding the maximum and minimum values.
- Write a C# program to compute the sum of squares of absolute differences between all elements in an array.
Go to:
PREV : Check Word Plural.
NEXT : Integer to String and Vice Versa.
C# Sharp Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.