C#: Program to take a number as input and print its multiplication table
Multiplication Table
Write a C# Sharp program that prints the multiplication table of a number as input.
In mathematics, a multiplication table is a mathematical table used to define a multiplication operation for an algebraic system.
C# Sharp multiplication table :
In mathematics, a multiplication table is a mathematical table used to define a multiplication operation for an algebraic system.
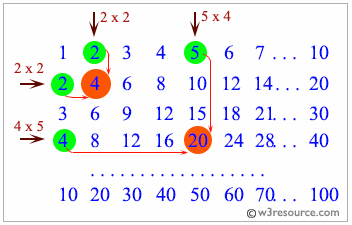
Sample Solution:
C# Sharp Code:
using System;
// This is the beginning of the Exercise8 class
public class Exercise8
{
// This is the main method where the program execution starts
public static void Main()
{
int x; // Variable to store the user input number
int result; // Variable to store the result of multiplication
Console.WriteLine("Enter a number:"); // Prompting the user to enter a number
x = Convert.ToInt32(Console.ReadLine()); // Reading the number entered by the user and converting it to an integer
// Loop through numbers from 1 to 10 to generate multiplication table for the entered number
result = x * 1;
Console.WriteLine("The table is : {0} x {1} = {2}", x, 1, result);
result = x * 2;
Console.WriteLine(" : {0} x {1} = {2}", x, 2, result);
result = x * 3;
Console.WriteLine(" : {0} x {1} = {2}", x, 3, result);
result = x * 4;
Console.WriteLine(" : {0} x {1} = {2}", x, 4, result);
result = x * 5;
Console.WriteLine(" : {0} x {1} = {2}", x, 5, result);
result = x * 6;
Console.WriteLine(" : {0} x {1} = {2}", x, 6, result);
result = x * 7;
Console.WriteLine(" : {0} x {1} = {2}", x, 7, result);
result = x * 8;
Console.WriteLine(" : {0} x {1} = {2}", x, 8, result);
result = x * 9;
Console.WriteLine(" : {0} x {1} = {2}", x, 9, result);
result = x * 10;
Console.WriteLine(" : {0} x {1} = {2}", x, 10, result);
}
}
Sample Output:
Enter a number: 5 The table is : 5 x 1 = 5 : 5 x 2 = 10 : 5 x 3 = 15 : 5 x 4 = 20 : 5 x 5 = 25 : 5 x 6 = 30 : 5 x 7 = 35 : 5 x 8 = 40 : 5 x 9 = 45 : 5 x 10 = 50
Flowchart:
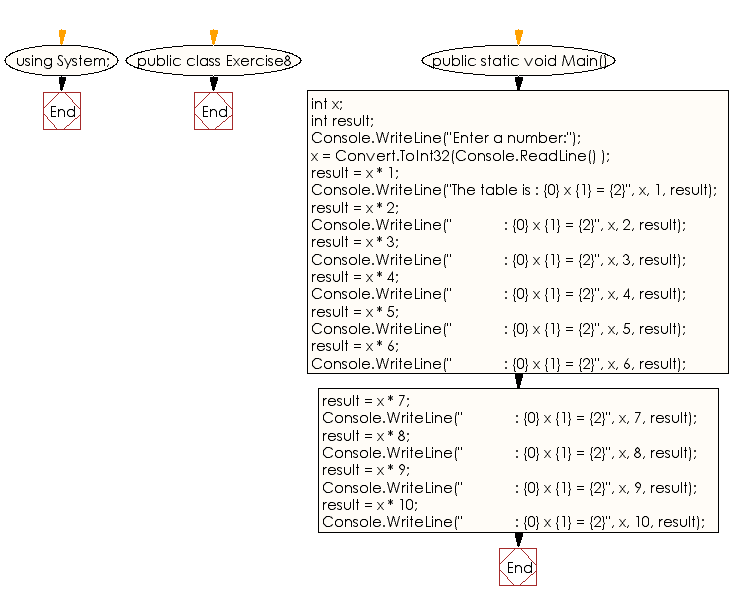
C# Sharp Code Editor:
Previous: Write a C# Sharp program to print on screen the output of adding, subtracting, multiplying and dividing of two numbers which will be entered by the user.
Next: Write a C# Sharp program that takes four numbers as input to calculate and print the average.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.