C#: Print the average of four numbers
Average of Four Numbers
Write a C# Sharp program that takes four numbers as input to calculate and print the average.
C# Sharp: Average of numbers
An average is the sum of a list of numbers divided by the number of numbers in the list. In mathematics and statistics, this would be called the arithmetic mean. The term "arithmetic mean" is preferred in some contexts in mathematics and statistics because it helps distinguish it from other means, such as the geometric mean and the harmonic mean.
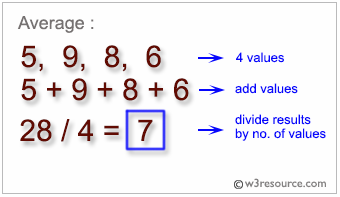
Sample Solution:
C# Sharp Code:
using System;
using System.IO;
// This is the beginning of the Exercise9 class
public class Exercise9
{
// This is the main method where the program execution starts
public static void Main()
{
// Declaration of variables to store four numbers
double number1, number2, number3, number4;
// Prompting the user to enter the first number
Console.Write("Enter the First number: ");
// Reading the first number entered by the user and converting it to a double
number1 = Convert.ToDouble(Console.ReadLine());
// Prompting the user to enter the second number
Console.Write("Enter the Second number: ");
// Reading the second number entered by the user and converting it to a double
number2 = Convert.ToDouble(Console.ReadLine());
// Prompting the user to enter the third number
Console.Write("Enter the third number: ");
// Reading the third number entered by the user and converting it to a double
number3 = Convert.ToDouble(Console.ReadLine());
// Prompting the user to enter the fourth number
Console.Write("Enter the fourth number: ");
// Reading the fourth number entered by the user and converting it to a double
number4 = Convert.ToDouble(Console.ReadLine());
// Calculating the average of the four numbers
double result = (number1 + number2 + number3 + number4) / 4;
// Displaying the average of the four numbers to the console
Console.WriteLine("The average of {0}, {1}, {2}, {3} is: {4} ",
number1, number2, number3, number4, result);
}
}
Sample Output:
Enter the First number: 17 Enter the Second number: 17 Enter the third number: 517 Enter the four number: 51 The average of 17, 17, 517, 51 is: 150.5
Flowchart:
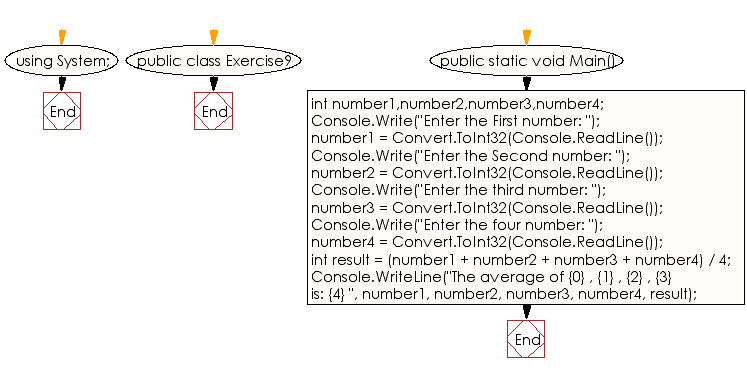
C# Sharp Code Editor:
Previous: Write a C# Sharp program that takes a number as input and print its multiplication table.
Next: Write a C# Sharp program to that takes three numbers(x,y,z) as input and print the output of (x+y)·z and x·y + y·z.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.