C#: Convert all the values of a given array of mixed values to string values
Convert Array Elements to Strings
Write a C# Sharp program to convert all the values of a given array of mixed values to string values.
Sample Solution:
C# Sharp Code:
using System;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Creating an array of objects to hold different types of values
object[] mixedArray = new object[5];
mixedArray[0] = 25; // Integer value
mixedArray[1] = "Anna"; // String value
mixedArray[2] = false; // Boolean value
mixedArray[3] = System.DateTime.Now; // Current date and time value
mixedArray[4] = 112.22; // Double value
// Displaying the original array elements and their types
Console.WriteLine("Printing original array elements and their types:");
for (int i = 0; i < mixedArray.Length; i++)
{
Console.WriteLine("Value-> " + mixedArray[i] + " :: Type-> " + mixedArray[i].GetType());
}
// Calling the test method to convert the array of objects to an array of strings
string[] new_nums = test(mixedArray);
// Displaying the converted array elements and their types
Console.WriteLine("\nPrinting array elements and their types after conversion:");
for (int i = 0; i < new_nums.Length; i++)
{
Console.WriteLine("Value-> " + new_nums[i] + " :: Type-> " + new_nums[i].GetType());
}
}
// Method to convert an array of objects to an array of strings
public static string[] test(object[] nums)
{
// Using Array.ConvertAll to convert each object to its string representation
return Array.ConvertAll(nums, x => x.ToString());
}
}
}
Sample Output:
Printing original array elements and their types: Value-> 25 :: Type-> System.Int32 Value-> Anna :: Type-> System.String Value-> False :: Type-> System.Boolean Value-> 4/15/2021 10:37:47 AM :: Type-> System.DateTime Value-> 112.22 :: Type-> System.Double Printing array elements and their types: Value-> 25 :: Type-> System.String Value-> Anna :: Type-> System.String Value-> False :: Type-> System.String Value-> 4/15/2021 10:37:47 AM :: Type-> System.String Value-> 112.22 :: Type-> System.String
Flowchart:
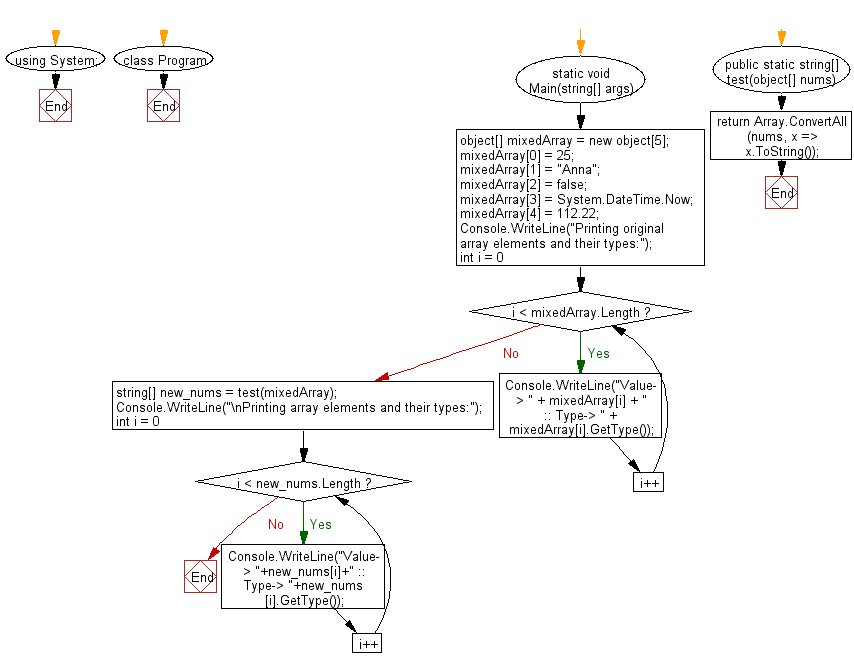
For more Practice: Solve these Related Problems:
- Write a C# program to convert all elements of a mixed array to their string representation and identify their original types.
- Write a C# program to convert a dictionary of mixed value types into a dictionary of string values.
- Write a C# program to serialize an array of objects of different types into a single JSON string.
- Write a C# program to convert each object in an object array to string and sort them alphabetically.
Go to:
PREV : Integer to String and Vice Versa.
NEXT : Check Swapped Two-Digit Number.
C# Sharp Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.