C#: Check whether a two digit given number is greater than its swap value
C# Sharp Basic: Exercise-81 with Solution
Check Swapped Two-Digit Number
Write a C# Sharp program to swap a two-digit number and check whether the given number is greater than its swap value.
Sample Solution:
C# Sharp Code:
using System;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Prompting the user to input an integer value
Console.WriteLine("Input an integer value:");
// Reading the input from the user and converting it to an integer
int n = Convert.ToInt32(Console.ReadLine());
// Checking if the given value is greater than or equal to its swap value
Console.WriteLine("Check whether the said value is greater than its swap value: " + test(n));
}
// Method to check if a value is greater than its swap value
public static bool test(int n)
{
// Calculating the swap value and comparing it with the original value
return (int)(n / 10) >= n % 10;
}
}
}
Sample Output:
Input an integer value: Check whether the said value is greater than its swap value: True
Flowchart:
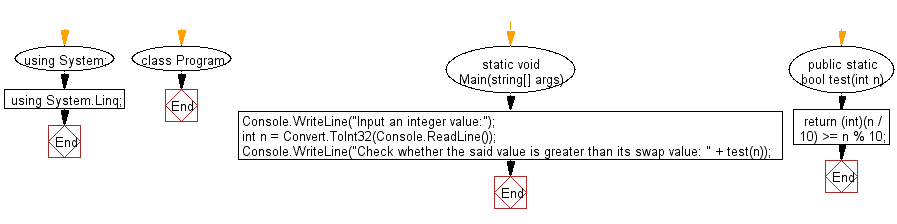
C# Sharp Code Editor:
Previous: Write a C# Sharp program to convert all the values of a given array of mixed values to string values.
Next: Write a C# Sharp program to remove all characters which are non-letters from a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/basic/csharp-basic-exercise-81.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics