C# Sharp Exercises: Check if a string is numeric or not
C# Sharp Basic: Exercise-97 with Solution
Check Numeric String
Write a C# Sharp program to check if a given string (floating point and negative numbers included) is numeric or not. Return True if the string is numeric, otherwise false.
Sample Data:
("123") -> True
("123.33") -> True
("33/33") -> False
("234234d2") -> False
Sample Solution:
C# Sharp Code:
using System;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Initializing different strings
string text = "123";
Console.WriteLine("Original string: " + text);
Console.WriteLine("Check if the said string is numeric or not! " + test(text));
text = "123.33";
Console.WriteLine("Original string: " + text);
Console.WriteLine("Check if the said string is numeric or not! " + test(text));
text = "33/33";
Console.WriteLine("Original string: " + text);
Console.WriteLine("Check if the said string is numeric or not! " + test(text));
text = "234234d2";
Console.WriteLine("Original string: " + text);
Console.WriteLine("Check if the said string is numeric or not! " + test(text));
}
// Method to check if a string is numeric
public static bool test(string text)
{
double Result; // Declare a variable to store the parsed result
// Try parsing the input string as a double
// If successful, the string is numeric, return true; otherwise, return false
return double.TryParse(text, out Result);
}
}
}
Sample Output:
Original string: 123 Check if the said string is numeric or not! True Original string: 123.33 Check if the said string is numeric or not! True Original string: 33/33 Check if the said string is numeric or not! False Original string: 234234d2 Check if the said string is numeric or not! False
Flowchart:
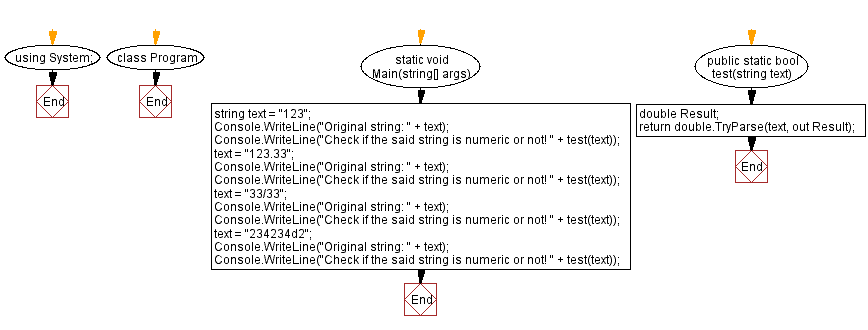
C# Sharp Code Editor:
Previous C# Sharp Exercise: String with same characters.
Next C# Sharp Exercise: Prime number in strictly descending decimal digit order.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/basic/csharp-basic-exercise-97.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics