C#: Prime number in strictly descending decimal digit order
Primes in Descending Order
Write a C# Sharp program to create and display all prime numbers in strictly descending decimal digit order.
Sample Data:
2, 3, 5, 7, 31, 41, 43, 53, 61, 71, 73, 83, 97, 421, 431.....
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
// Method to check if a number is prime
public static bool IsPrime(uint n)
{
if (n <= 1)
{
return false;
}
int ctr = 0;
for (int i = 1; i <= n; i++)
{
if (n % i == 0)
{
ctr++;
}
if (ctr > 2)
{
return false;
}
}
return true;
}
static void Main(string[] args)
{
uint z = 0; // Counter variable for prime numbers
int nc; // Variable to hold the count of numbers
// Array of prime numbers
var p = new uint[] { 1, 2, 3, 4, 5, 6, 7, 8, 9 };
var nxt = new uint[128]; // Array for next potential prime numbers
while (true)
{
nc = 0; // Reset the count of numbers
// Loop through each number in the array of prime numbers
foreach (var x in p)
{
// Check if the number is prime and display it
if (IsPrime(x))
Console.Write("{0,8}{1}", x, ++z % 5 == 0 ? "\n" : " ");
// Generate next potential prime numbers
for (uint y = x * 10, l = x % 10 + y++; y < l; y++)
nxt[nc++] = y;
}
// Check if there are more than one potential prime numbers
if (nc > 1)
{
// Resize the prime number array and copy next potential primes
Array.Resize(ref p, nc);
Array.Copy(nxt, p, nc);
}
else
{
// Break the loop if there are no more potential primes
break;
}
}
// Output the number of descending primes found
Console.WriteLine("\n{0} descending primes found", z);
}
}
}
Sample Output:
2 3 5 7 31 41 43 53 61 71 73 83 97 421 431 521 541 631 641 643 653 743 751 761 821 853 863 941 953 971 983 5431 6421 6521 7321 7541 7621 7643 8431 8521 8543 8641 8731 8741 8753 8761 9421 9431 9521 9631 9643 9721 9743 9851 9871 75431 76421 76541 76543 86531 87421 87541 87631 87641 87643 94321 96431 97651 98321 98543 98621 98641 98731 764321 865321 876431 975421 986543 987541 987631 8764321 8765321 9754321 9875321 97654321
Flowchart:
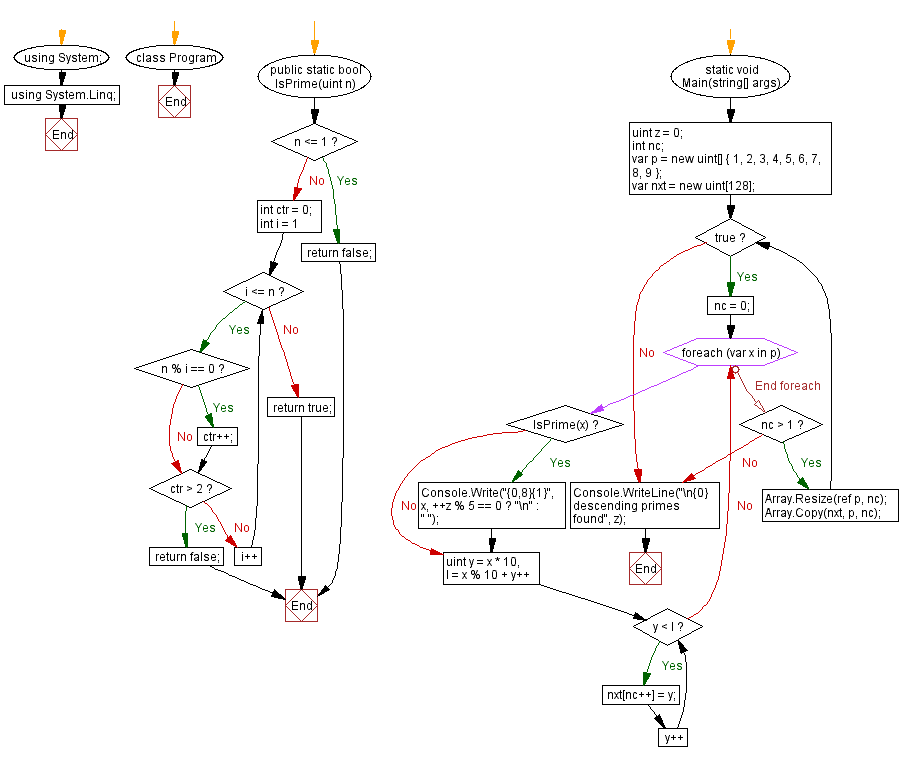
For more Practice: Solve these Related Problems:
- Write a C# program to generate descending prime numbers with digits in strictly descending order.
- Write a C# program to list primes less than a given number whose digits are in descending order.
- Write a C# program to find descending digit primes with exactly 3 digits.
- Write a C# program to count how many descending digit primes exist below 10,000.
Go to:
PREV : Check Numeric String.
NEXT : Primes in Ascending Order.
C# Sharp Code Editor:
What is the difficulty level of this exercise?